答辩问使用spring boot和 vue如何实现轮播图
时间: 2023-07-15 12:10:12 浏览: 55
使用Spring Boot和Vue实现轮播图可以通过以下步骤:
1. 在Spring Boot中,创建一个RESTful API来获取轮播图的数据,例如图片URL、标题等。可以使用Spring MVC或Spring WebFlux来实现。
2. 在Vue中,使用第三方插件如vue-awesome-swiper或element-ui的Carousel组件来实现轮播图。
3. 在Vue中,使用Axios或Fetch等库来调用RESTful API获取轮播图数据。
4. 在Vue组件中,将获取到的轮播图数据绑定到Carousel组件中。
5. 配置Carousel组件的属性,如轮播间隔时间、是否自动播放、是否显示分页等。
6. 根据需要,可以添加一些特效,如淡入淡出、滑动等。
7. 最后,在Vue组件中将Carousel组件渲染到页面中。
总之,通过以上步骤,可以很方便地在Spring Boot和Vue中实现轮播图。
相关问题
答辩问使用spring boot和 vue如何使用element组件实现轮播图
使用 Spring Boot 和 Vue,可以通过以下步骤实现轮播图:
1. 在 Spring Boot 中创建一个 RESTful API 接口,用于获取轮播图的数据。
2. 在 Vue 中使用 axios 或者其他 HTTP 库,调用该接口获取轮播图数据。
3. 在 Vue 中使用 Element 组件库中的 Carousel 组件来实现轮播图的展示。Carousel 组件支持自动轮播,可以根据获取到的数据动态渲染轮播图。
具体实现步骤如下:
1. 在 Spring Boot 中创建一个 RESTful API 接口,例如:
```java
@RestController
@RequestMapping("/api")
public class CarouselController {
@GetMapping("/carousel")
public List<Carousel> getCarousel() {
// 获取轮播图数据
List<Carousel> carouselList = new ArrayList<>();
carouselList.add(new Carousel(1, "https://example.com/image1.jpg"));
carouselList.add(new Carousel(2, "https://example.com/image2.jpg"));
carouselList.add(new Carousel(3, "https://example.com/image3.jpg"));
return carouselList;
}
}
```
2. 在 Vue 中使用 axios 或者其他 HTTP 库,调用该接口获取轮播图数据,例如:
```javascript
<script>
import axios from 'axios';
export default {
data() {
return {
carouselList: [],
};
},
mounted() {
this.getCarousel();
},
methods: {
getCarousel() {
axios.get('/api/carousel').then((response) => {
this.carouselList = response.data;
});
},
},
};
</script>
```
3. 在 Vue 中使用 Element 组件库中的 Carousel 组件来实现轮播图的展示,例如:
```html
<template>
<el-carousel :interval="5000">
<el-carousel-item v-for="carousel in carouselList" :key="carousel.id">
<img :src="carousel.imageUrl" alt="carousel">
</el-carousel-item>
</el-carousel>
</template>
```
这样,就可以通过 Spring Boot 和 Vue 实现轮播图了。
答辩问使用spring boot和 vue如何实现前后端分离
使用Spring Boot和Vue实现前后端分离,可以按照以下步骤进行:
1. 后端使用Spring Boot框架搭建RESTful API服务,提供数据接口;
2. 前端使用Vue框架编写页面,通过Ajax等方式请求后端提供的数据接口;
3. 前端页面和后端数据接口交互采用JSON格式数据,使得数据传输更加高效;
4. 前端代码和后端代码分别部署在不同的服务器上,通过跨域的方式实现数据交互。
具体实现步骤如下:
1. 后端使用Spring Boot框架搭建RESTful API服务
可以使用Spring Boot框架来搭建后端服务,使用Spring MVC来构建RESTful API。可以使用Spring Boot的starter包,来简化Spring MVC的配置。
2. 前端使用Vue框架编写页面
可以使用Vue框架编写前端页面。Vue提供了一套MVVM模式的数据绑定和组件化的开发方式,可以更加方便地编写前端页面。
3. 前端页面和后端数据接口交互采用JSON格式数据
前端页面通过Ajax等方式请求后端提供的数据接口,后端返回JSON格式的数据。这样可以使得数据传输更加高效。
4. 前端代码和后端代码分别部署在不同的服务器上
可以将前端代码和后端代码部署在不同的服务器上,通过跨域的方式实现数据交互。可以设置CORS(跨域资源共享)来实现跨域访问。
总的来说,使用Spring Boot和Vue实现前后端分离,可以提高开发效率和系统性能,同时也可以更好地实现前后端分离。
相关推荐
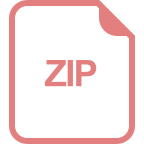
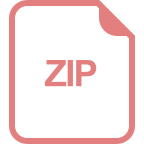










