答辩问使用spring boot和 vue如何实现在够买商品时加减数量
时间: 2023-06-26 16:09:16 浏览: 109
在使用Spring Boot和Vue实现购买商品时加减数量,可以通过以下步骤:
1. 在Vue中,先定义一个变量来保存商品数量,例如:
```
<template>
<div>
<button @click="decrement">-</button>
<span>{{ quantity }}</span>
<button @click="increment">+</button>
</div>
</template>
<script>
export default {
data() {
return {
quantity: 1 // 商品数量初始值为1
}
},
methods: {
decrement() {
if (this.quantity > 1) { // 避免商品数量小于1
this.quantity--
}
},
increment() {
this.quantity++
}
}
}
</script>
```
2. 在Spring Boot中,定义一个接口来处理商品数量的增加和减少:
```
@RestController
@RequestMapping("/api")
public class CartController {
@PostMapping("/cart/add")
public void addToCart(@RequestParam("productId") Long productId, @RequestParam("quantity") int quantity) {
// 将商品添加到购物车
}
@PostMapping("/cart/update")
public void updateCart(@RequestParam("productId") Long productId, @RequestParam("quantity") int quantity) {
// 更新购物车中商品的数量
}
@PostMapping("/cart/remove")
public void removeFromCart(@RequestParam("productId") Long productId) {
// 从购物车中删除商品
}
}
```
3. 在Vue中,通过axios发送请求调用Spring Boot中的接口:
```
import axios from 'axios'
export default {
methods: {
async addToCart(productId, quantity) {
await axios.post('/api/cart/add', { productId, quantity })
},
async updateCart(productId, quantity) {
await axios.post('/api/cart/update', { productId, quantity })
},
async removeFromCart(productId) {
await axios.post('/api/cart/remove', { productId })
}
}
}
```
通过以上步骤,就可以实现在购买商品时加减数量了。在Vue中,用户点击加减按钮时,会更新商品数量的变量;在Spring Boot中,接口会接收前端发送的请求,然后对购物车中商品的数量进行相应的操作。
阅读全文
相关推荐
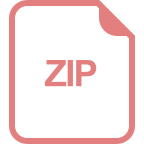












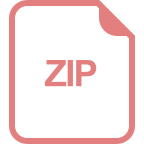