设有字符串S及长度为n的字符型一维数组a,编写一个函数,统计a中每个字符在字符串S中出现的次数。要求该函数以S、a、n为形参,一维整型数组为返回值。使用c语言
时间: 2024-10-08 17:01:48 浏览: 20
在C语言中,你可以创建一个这样的函数,名为`countCharsInString`,它接受三个参数:`const char* S`(字符串S的指针)、`char a[]`(字符数组a)和`int n`(数组a的长度),然后返回一个一维整数数组,表示每个字符在`S`中出现的次数。以下是函数的一个实现示例:
```c
#include <stdio.h>
#include <string.h>
// 函数声明
typedef struct {
int count;
char c;
} CountResult;
CountResult* countCharsInString(const char* S, char a[], int n) {
// 初始化结果数组
CountResult results[n];
for (int i = 0; i < n; i++) {
results[i].count = 0;
}
// 遍历S中的每一个字符
for (const char* s_char = S; *s_char != '\0'; ++s_char) {
// 对于数组中的每个字符,检查它是否等于当前S中的字符
for (int i = 0; i < n; i++) {
if (a[i] == *s_char) {
// 如果相等,增加计数
results[i].count++;
break; // 只需找到一次就跳过后续元素
}
}
}
return results;
}
void printCounts(CountResult* counts, int n) {
for (int i = 0; i < n; i++) {
printf("Character '%c': %d times\n", counts[i].c, counts[i].count);
}
}
int main() {
char a[] = {'h', 'e', 'l', 'l', 'o'};
const char* S = "hello";
int n = sizeof(a) / sizeof(a[0]);
CountResult* results = countCharsInString(S, a, n);
printCounts(results, n);
free(results); // 释放内存
return 0;
}
```
在这个例子中,`countCharsInString`函数首先初始化了一个结果数组,然后遍历`S`中的每个字符并查找对应位置在`a`中的字符,如果找到则计数。最后,`printCounts`函数用于打印每个字符及其出现次数。
相关推荐
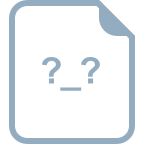
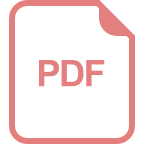
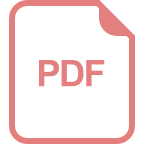
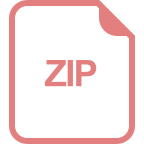
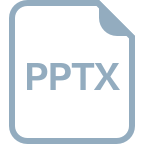
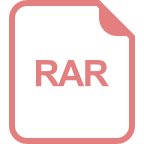
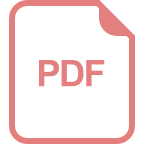
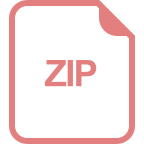
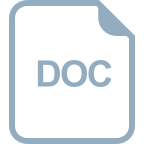
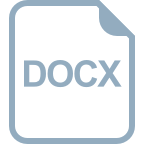
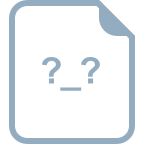
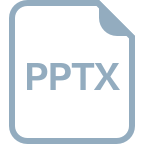
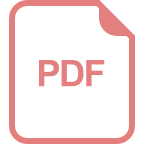
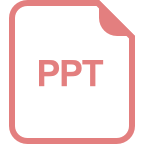
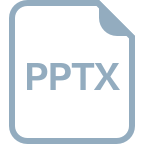
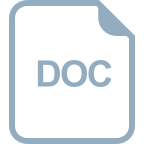
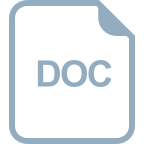
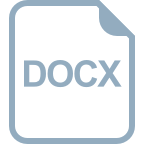
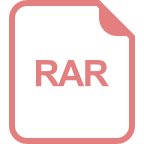