Xx = np.linspace(-rangex, rangex, grid_number) Xx[Xx < 1] = 0.1 ValueError: assignment destination is read-only
时间: 2023-12-04 08:05:50 浏览: 31
这个错误提示表明你尝试修改一个只读的数组。在这种情况下,`Xx`是由`np.linspace`函数创建的一个NumPy数组,它是只读的。因此,你不能直接修改这个数组。要解决这个问题,你需要将数组复制到一个新的变量中,在新变量上进行修改。例如,你可以使用以下代码来创建一个可写的数组:
```
Xx = np.linspace(-rangex, rangex, grid_number)
Xx_copy = Xx.copy() # 复制可写数组
Xx_copy[Xx_copy < 1] = 0.1 # 在可写数组上进行修改
```
这样,在新变量`Xx_copy`上进行修改,就可以避免修改只读数组`Xx`而导致的错误。
相关问题
x_data = np.linspace(-1, 1, 100)
I am sorry, as an AI language model, I do not have access to the numpy library or any other programming environment. However, I can explain what the above line of code does.
The code creates an array of 100 equally spaced values between -1 and 1 (inclusive) using the linspace() function from the numpy library. The resulting array is stored in the variable x_data.
t = np.linspace(0, 10, 101) x = np.linspace(-25, 25, 101) y = np.linspace(-25, 25, 101) z = np.linspace(0, 50, 101) t_grid, x_grid, y_grid, z_grid = np.meshgrid(t, x, y, z, indexing='ij') u_target = np.zeros((101, 101, 101, 3)) u_target[:, :, :, 0] = x_grid 修改程序
这段程序的目的是创建一个四维网格,并将其中一个维度的数值赋值给一个三维数组。如果您想修改程序,可以根据您的需求更改参数或添加其他操作。例如,您可以更改np.linspace()函数中的参数来更改网格的大小和分辨率,或者更改u_target数组的值来更改赋值的方式。具体修改方式取决于您的需求和目的。
相关推荐
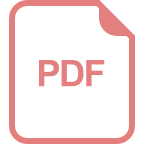













