Input type (torch.cuda.FloatTensor) , weight type (torch.FloatTensor) 怎么把input变成torch.floattensor
时间: 2023-09-24 22:03:52 浏览: 77
要将输入张量(input)的类型从 torch.cuda.FloatTensor 更改为 torch.FloatTensor,你可以使用 `.float()` 方法将其转换为 float 类型的张量。
以下是一种可能的解决方法:
```python
import torch
# 假设你有一个名为 input_tensor 的张量
input_tensor = input_tensor.float() # 将输入张量转换为 float 类型的张量
# 然后使用转换后的张量进行操作
output = F.conv2d(input_tensor, weight, bias, stride)
```
在上述代码中,`.float()` 方法将输入张量转换为 float 类型的张量。然后,你可以使用转换后的张量进行卷积操作。
请注意,这样做会在 CPU 上执行操作。如果你想在 GPU 上执行操作,你需要将张量移动到 GPU 上:
```python
input_tensor = input_tensor.float().to(torch.device("cuda")) # 将输入张量转换为 float 类型的张量,并将其移动到 GPU
```
确保你的硬件和软件环境支持 GPU 计算,并正确配置了 CUDA 和其他相关的库。如果你没有可用的 GPU,你可以考虑在 CPU 上运行相关的函数或使用其他适合 CPU 的替代函数。
相关问题
RuntimeError: Input type (torch.cuda.FloatTensor) and weight type (torch.cuda.HalfTensor) should be the same
这个错误通常是因为输入张量的数据类型与模型权重张量的数据类型不匹配导致的。在这种情况下,输入张量的数据类型是`torch.cuda.FloatTensor`,而模型权重张量的数据类型是`torch.cuda.HalfTensor`,它们不匹配。
要解决这个问题,你需要确保输入张量和模型权重张量的数据类型相同。你可以使用`torch.Tensor.type()`方法将输入张量转换为与模型权重张量相同的数据类型,或者使用`torch.nn.Module.half()`方法将模型权重张量转换为与输入张量相同的数据类型,这取决于你的具体情况。
如何解决Input type (torch.cuda.FloatTensor) and weight type (torch.cuda.HalfTensor) should be the same的错误
当你遇到 "Input type (torch.cuda.FloatTensor) and weight type (torch.cuda.HalfTensor)" 这样的错误时,它通常发生在PyTorch中,当操作的数据类型不匹配时。这是因为神经网络模型中的权重(weight)通常是存储为半精度浮点数(HalfTensor),而输入数据(如张量)可能是单精度浮点数(FloatTensor)。要解决这个问题,你需要将输入转换成半精度:
1. **数据预处理**: 如果可能的话,在加载或创建张量之前,确保将其转换为half类型。例如,你可以这样做:
```python
input_data = input_data.type(torch.half)
```
2. **设置层的 dtype**: 对于某些深度学习库,特别是那些允许设置层内部张量类型的,可以在构建层时指定dtype。例如,在PyTorch的`nn.Module`中,可以这样创建一个半精度的线性层:
```python
linear_layer = nn.Linear(in_features, out_features, bias=True, dtype=torch.half)
```
3. **后处理**: 在完成运算后,如果需要返回原始精度的输出,可以在计算结束后再转换回单精度:
```python
output = output.float()
```
**相关问题--:**
1. 半精度浮点数在深度学习中有何优势?
2. 在训练过程中为什么经常使用半精度而不是单精度?
3. 如果我在测试阶段也遇到了这个错误,如何处理?
阅读全文
相关推荐
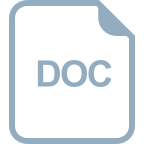
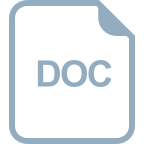
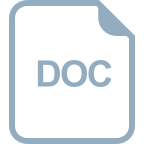

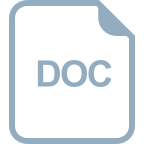
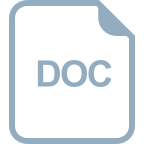
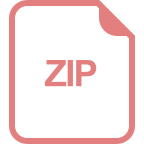
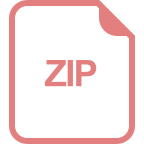
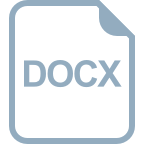
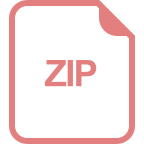
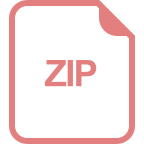
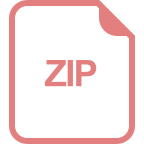
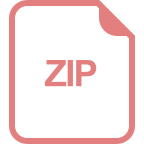
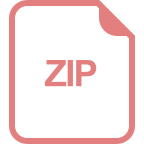