请你把MobileNetV3_Small 代码模型写出来
时间: 2024-03-26 13:35:27 浏览: 15
好的,这里是 MobileNetV3_Small 的代码实现:
```
import torch
import torch.nn as nn
class ConvBNReLU(nn.Sequential):
"""Convolutional layer with batch normalization and ReLU activation."""
def __init__(self, in_planes, out_planes, kernel_size=3, stride=1, groups=1):
padding = (kernel_size - 1) // 2
super(ConvBNReLU, self).__init__(
nn.Conv2d(in_planes, out_planes, kernel_size, stride, padding, groups=groups, bias=False),
nn.BatchNorm2d(out_planes),
nn.ReLU6(inplace=True)
)
class InvertedResidual(nn.Module):
"""Inverted residual block."""
def __init__(self, inp, oup, stride, expand_ratio):
super(InvertedResidual, self).__init__()
hidden_dim = round(inp * expand_ratio)
self.use_res_connect = stride == 1 and inp == oup
layers = []
if expand_ratio != 1:
# pointwise
layers.append(ConvBNReLU(inp, hidden_dim, kernel_size=1))
layers.extend([
# depthwise
ConvBNReLU(hidden_dim, hidden_dim, stride=stride, groups=hidden_dim),
# pointwise-linear
nn.Conv2d(hidden_dim, oup, 1, 1, 0, bias=False),
nn.BatchNorm2d(oup),
])
self.conv = nn.Sequential(*layers)
def forward(self, x):
if self.use_res_connect:
return x + self.conv(x)
else:
return self.conv(x)
class MobileNetv3_small(nn.Module):
"""MobileNetV3_Small model."""
def __init__(self, num_classes=1000, width_mult=1.0):
super(MobileNetv3_small, self).__init__()
# setting of inverted residual blocks
self.cfgs = [
# k, t, c, SE, s
[3, 16, 16, 0, 1],
[3, 72, 24, 0, 2],
[3, 88, 24, 0, 1],
[5, 96, 40, 1, 2],
[5, 240, 40, 1, 1],
[5, 240, 40, 1, 1],
[5, 120, 48, 1, 1],
[5, 144, 48, 1, 1],
[5, 288, 96, 1, 2],
[5, 576, 96, 1, 1],
[5, 576, 96, 1, 1],
]
# building first layer
input_channel = int(16 * width_mult)
last_channel = int(576 * width_mult) if width_mult > 1.0 else 576
self.features = [ConvBNReLU(3, input_channel, stride=2)]
# building inverted residual blocks
for k, exp_size, c, se, s in self.cfgs:
output_channel = int(c * width_mult)
self.features.append(InvertedResidual(input_channel, output_channel, stride=s, expand_ratio=exp_size/ input_channel))
input_channel = output_channel
# building last several layers
self.features.append(ConvBNReLU(input_channel, last_channel, kernel_size=1))
self.features.append(nn.AdaptiveAvgPool2d((1, 1)))
self.features.append(nn.Conv2d(last_channel, 1024, kernel_size=1, stride=1, padding=0, bias=False))
self.features.append(nn.Hardswish())
self.features.append(nn.Conv2d(1024, num_classes, kernel_size=1, stride=1, padding=0, bias=True))
self.features = nn.Sequential(*self.features)
def forward(self, x):
x = self.features(x)
x = x.view(x.size(0), -1)
return x
```
这个实现中包含了 MobileNetV3_Small 模型中使用的 Inverted Residual Block 和 ConvBNReLU 等基本组件。同时,在实现模型的过程中,作者还考虑到了模型的可配置性,可以通过调整宽度因子 `width_mult` 来控制模型的宽度。
相关推荐
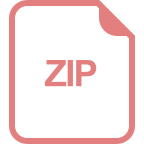
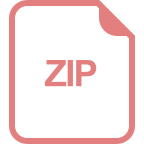














