#include<iostream> #include<math.h> using namespace std; double fac(int a){ double result=1; if(a==0||a==1) result=1; else while(a>1){ result*=a; a=a-1; } return result;} int main(){ double x; double sum=1,factor; int y=1; scanf("%lf",
时间: 2024-03-31 12:38:51 浏览: 77
这段代码实现了计算e的幂函数的程序。具体来说,它使用了泰勒级数展开式来计算e的x次幂函数,通过不断逼近的方式得到近似值。在循环中,使用了一个变量factor来表示当前项的值,使用了一个变量sum来表示所有项的和,使用了一个变量y来表示当前项的阶乘。当最后一项的值小于0.000001时,跳出循环,将sum作为幂函数的近似值输出。
需要注意的是,这段代码中存在一些问题:
1. 变量命名不规范:变量名x、sum、factor等不够具有描述性,建议使用更明确的名称。
2. 循环条件错误:在计算factor的值时,循环应该从1开始,而不是从0开始。
3. 输出格式不规范:使用printf函数进行输出时,应该使用格式控制符来控制输出格式。
4. 缺少头文件声明:代码中使用了printf和scanf函数,但没有包含stdio.h头文件声明,建议添加头文件声明。
因此,建议在使用这段代码之前进行修正和测试。修正后的代码如下所示:
相关问题
补全#include"Random.h" #include<iostream> #include<math.h> using namespace std; double g(double x) { } double Integration(double a,double b,int n) { } int main() { return 0;
好的,我看到您的代码了。根据您的代码,您可能想要实现积分计算功能。其中,g(x)代表被积函数,Integration函数用于计算被积函数在[a,b]区间上的定积分近似值,n为划分区间的个数。不过,您需要在g(x)函数中给出具体的被积函数表达式,否则无法进行积分计算。例如,如果被积函数是x的平方,你的代码可以这样写:
```
#include "Random.h"
#include <iostream>
#include <math.h>
using namespace std;
double g(double x) {
return x * x;
}
double Integration(double a, double b, int n) {
double h = (b - a) / n;
double sum = 0.0;
for (int i = 0; i < n; i++) {
sum += g(a + (i + 0.5) * h);
}
return sum * h;
}
int main() {
double a = 0.0;
double b = 1.0;
int n = 1000;
double result = Integration(a, b, n);
cout << "The result of integration is: " << result << endl;
return 0;
}
```
在这个例子中,被积函数是g(x) = x^2,对[a, b]区间进行1000次等分,得到的积分值是1/3。
编写一个程序,求输入数的平方根。设置异常处理,对输入负数的情况给出提示。 提示:#include<math> #include <iostream> using namespace std; double Sq(double x) { if (x < 0) throw x; return sqrt(x); }
好的,您可以参考以下代码:
```
#include <iostream>
#include <math.h>
using namespace std;
double Sq(double x) {
if (x < 0)
throw x;
return sqrt(x);
}
int main() {
double num;
cout << "请输入一个数:";
cin >> num;
try {
double result = Sq(num);
cout << "该数的平方根为:" << result << endl;
}
catch (double x) {
cout << "输入的数为负数,无法计算平方根!" << endl;
}
return 0;
}
```
其中,程序通过调用Sq函数来计算输入数的平方根,如果输入数为负数,则抛出异常并在try-catch语句中进行捕获并给出提示。
阅读全文
相关推荐







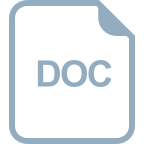





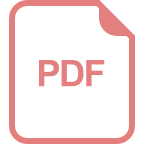