请写出折半查找的算法,请写出尾插法建立链表的算法
时间: 2024-10-12 11:16:50 浏览: 23
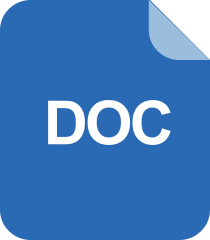
请写出对有序表进行折半查找的非递归算法.doc
当然,我很乐意为您介绍。
### 折半查找算法(Binary Search)
```python
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1 # 如果未找到目标,返回-1表示不存在
# 示例:
arr = [1, 3, 5, 7, 9]
target = 5
result = binary_search(arr, target)
if result != -1:
print(f"找到了,索引为 {result}")
else:
print("没找到")
```
### 尾插法建立链表(Tail Insertion Algorithm for LinkedLists)
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
def tail_insert(head, new_data):
if not head:
return Node(new_data)
current = head
while current.next:
current = current.next
current.next = Node(new_data)
return head
# 示例:
head = Node(1)
head.next = Node(2)
head.next.next = Node(3)
new_data = 4
tail_head = tail_insert(head, new_data)
```
在这个尾插法中,我们首先检查链表是否为空,然后从头开始迭代直到找到最后一个节点,将其`next`指针指向新插入的节点。
阅读全文
相关推荐
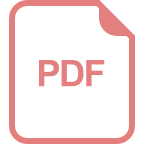
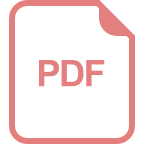
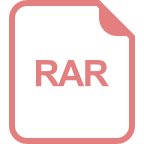
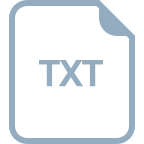
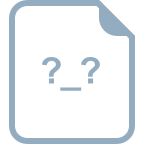
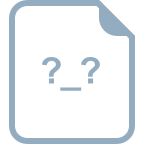
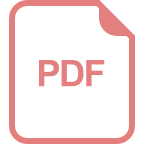
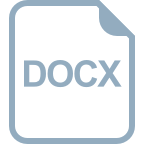
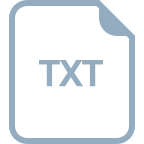
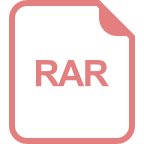
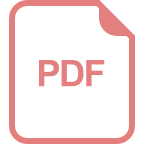
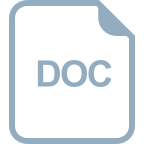
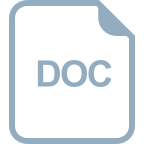
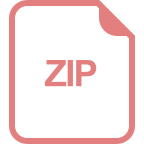