将Numpy ndarray数据转换为 PNG图片格式,然后用base64编码
时间: 2024-05-02 17:20:13 浏览: 93
可以使用Pillow库,先将ndarray数据转换为PIL.Image对象,再将其保存为PNG格式,最后使用base64编码。以下是示例代码:
```python
import numpy as np
from PIL import Image
import base64
# 生成一个随机的ndarray数据
data = np.random.rand(100, 100) * 255
data = data.astype('uint8')
# 将ndarray数据转换为PIL.Image对象
img = Image.fromarray(data)
# 保存为PNG格式
img.save('test.png')
# 打开文件并读取二进制数据
with open('test.png', 'rb') as f:
png_data = f.read()
# 将二进制数据进行base64编码
png_base64 = base64.b64encode(png_data).decode('utf-8')
print(png_base64)
```
输出结果为经过base64编码的PNG图片数据字符串。
相关问题
将Numpy ndarray数据转换为 JPG 图片格式,然后用base64编码
可以使用Python Pillow库来实现。具体步骤如下:
1. 导入Pillow库:
```
from PIL import Image
import numpy as np
import base64
```
2. 将numpy ndarray数据转换为Pillow Image对象:
```
data = np.random.random((256, 256)) # 示例随机数据
img = Image.fromarray(np.uint8(data*255))
```
3. 将Pillow Image对象保存为JPG格式文件,并读取文件数据:
```
with open("image.jpg", "wb") as f:
img.save(f, format='JPEG')
with open("image.jpg", "rb") as f:
jpg_data = f.read()
```
4. 将JPG文件数据进行base64编码:
```
base64_data = base64.b64encode(jpg_data)
```
最终得到的base64_data即为转换后的结果。注意:如果数据量较大,base64编码后可能会超出URL或数据URI的长度限制,需要进行分片处理。
将Numpy 【480,640,3】的 ndarray数据转换为 JPG 图片格式,然后用base64编码
可以使用Python中的PIL库来进行转换和编码。具体的代码如下:
```python
import numpy as np
from PIL import Image
import base64
# 将 numpy 数组转换为图像
arr = np.random.randint(0, 255, size=(480, 640, 3)).astype(np.uint8)
img = Image.fromarray(arr)
# 将图像转换为 JPG 格式
buffer = io.BytesIO()
img.save(buffer, format='JPEG')
jpg_data = buffer.getvalue()
# 使用 base64 对 JPG 数据进行编码
base64_data = base64.b64encode(jpg_data)
```
其中,首先将 numpy 数组转换为图像,然后使用 PIL 库的 save 方法将其保存为 JPG 格式。接着,使用 base64 库的 b64encode 方法对 JPG 数据进行编码,得到 base64 格式的数据。
阅读全文
相关推荐
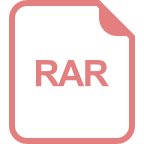













