如何将列表转换成张量
时间: 2024-02-27 08:51:45 浏览: 31
要将一个列表转换成张量,可以使用Python的NumPy库或者PyTorch库。在NumPy中,可以使用`numpy.array()`函数将列表转换成NumPy数组,然后使用`numpy.asarray()`函数将NumPy数组转换成张量。示例如下:
```python
import numpy as np
import torch
# 将列表转换成NumPy数组
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list)
# 将NumPy数组转换成张量
my_tensor = torch.from_numpy(my_array)
print(my_tensor)
```
在PyTorch中,可以直接使用`torch.Tensor()`函数将列表转换成张量。示例如下:
```python
import torch
# 将列表转换成张量
my_list = [1, 2, 3, 4, 5]
my_tensor = torch.Tensor(my_list)
print(my_tensor)
```
需要注意的是,在PyTorch中,`torch.Tensor()`函数默认返回的是FloatTensor类型的张量。如果需要返回其他类型的张量,可以使用`torch.LongTensor()`、`torch.IntTensor()`等函数。
相关问题
将列表转换为张量并输出张量的形状
好的,我假设您使用的是 Python 语言和 PyTorch 库。以下是将列表转换为张量并输出张量形状的代码示例:
```python
import torch
# 假设您有一个包含 10 个整数的列表
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# 将列表转换为 1 维张量
my_tensor = torch.tensor(my_list)
# 输出张量的形状
print(my_tensor.shape)
```
输出结果应该类似于:
```
torch.Size([10])
```
这表示您的张量是一个具有 10 个元素的一维张量。
pytorch中将列表元素转换为张量
可以使用`torch.Tensor()`函数将列表元素转换为张量。例如,将列表`[1, 2, 3]`转换为张量的代码如下:
```
import torch
my_list = [1, 2, 3]
my_tensor = torch.Tensor(my_list)
print(my_tensor)
```
输出结果为:
```
tensor([1., 2., 3.])
```
注意,由于`torch.Tensor()`函数默认创建的是`FloatTensor`类型的张量,因此转换后的元素类型为浮点数。如果需要创建其他类型的张量,可以使用`torch.LongTensor()`、`torch.ByteTensor()`等函数。
相关推荐
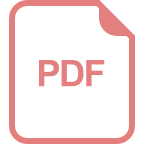












