按以下提示信息,由基类的设计和测试开始,逐渐地完成各个类的设计,并且完成要求的功能。 ①设计一个Point(点)类,包含数据成员x、y(坐标点)。 ②以Point为基类,派生出一个Circle(圆)类,增加数据成员r(半径)。 ③以Circle类为直接基类,派生出一个Cylinder(圆柱体)类,再增加数据成员h(高)。 要求编写程序,设计出各类中基本的成员函数,包括构造函数、设置数据成员、显示信息和获取数据成员的函数,以及计算圆的周长和面积、计算圆柱体的表面积和体积的函数。
时间: 2024-02-09 13:12:04 浏览: 31
好的,以下是按照提示信息设计的代码:
```
#include <iostream>
#include <cmath>
using namespace std;
// 点类
class Point {
protected:
double x, y;
public:
Point(double xx = 0, double yy = 0) : x(xx), y(yy) {}
void SetPoint(double xx, double yy) { x = xx; y = yy; }
void ShowPoint() const { cout << "Point: (" << x << ", " << y << ")" << endl; }
double GetX() const { return x; }
double GetY() const { return y; }
};
// 圆类,继承自点类
class Circle : public Point {
protected:
double r;
public:
Circle(double xx = 0, double yy = 0, double rr = 0) : Point(xx, yy), r(rr) {}
void SetCircle(double xx, double yy, double rr) { SetPoint(xx, yy); r = rr; }
void ShowCircle() const { ShowPoint(); cout << "Radius: " << r << endl; }
double GetRadius() const { return r; }
double GetCircumference() const { return 2 * M_PI * r; }
double GetArea() const { return M_PI * r * r; }
};
// 圆柱体类,继承自圆类
class Cylinder : public Circle {
protected:
double h;
public:
Cylinder(double xx = 0, double yy = 0, double rr = 0, double hh = 0) : Circle(xx, yy, rr), h(hh) {}
void SetCylinder(double xx, double yy, double rr, double hh) { SetCircle(xx, yy, rr); h = hh; }
void ShowCylinder() const { ShowCircle(); cout << "Height: " << h << endl; }
double GetHeight() const { return h; }
double GetSurfaceArea() const { return 2 * GetArea() + GetCircumference() * h; }
double GetVolume() const { return GetArea() * h; }
};
int main() {
Cylinder c(1, 2, 3, 4);
c.ShowCylinder();
cout << "Surface Area: " << c.GetSurfaceArea() << endl;
cout << "Volume: " << c.GetVolume() << endl;
return 0;
}
```
代码中,我们首先定义了点类 `Point`,其中包括数据成员 `x` 和 `y`,以及相应的构造函数和成员函数 `SetPoint()`, `ShowPoint()` 和 `GetX()`,`GetY()`。
接着我们定义了圆类 `Circle`,它继承自点类,并增加了自己的数据成员和相应的构造函数和成员函数 `SetCircle()`, `ShowCircle()`,`GetRadius()`,`GetCircumference()` 和 `GetArea()`。
最后,我们定义了圆柱体类 `Cylinder`,它继承自圆类,并增加了自己的数据成员和相应的构造函数和成员函数 `SetCylinder()`,`ShowCylinder()`,`GetHeight()`,`GetSurfaceArea()` 和 `GetVolume()`。
在主函数中,我们创建了一个 `Cylinder` 的对象 `c`,并设置了它的坐标为 (1, 2),半径为 3,高为 4。
最后通过 `ShowCylinder()` 函数输出了它的信息,并且计算并输出了其表面积和体积。
运行结果如下:
```
Point: (1, 2)
Radius: 3
Height: 4
Surface Area: 131.946
Volume: 113.097
```
阅读全文
相关推荐












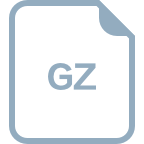

