编写一个生日提醒程序,用户需要输入自己的生日(格式为月/日/年,其中月,日为1-2位整数,年为1-4位整数)。程序将自动将日期转换为yyyymmdd的形式,并将其储存起来。
时间: 2024-09-13 17:16:39 浏览: 50
编写一个生日提醒程序的步骤可以分为以下几个部分:
1. 用户输入:首先,创建一个函数或方法,提示用户输入他们的生日,按照“月/日/年”的格式,并确保输入的是有效的数字范围。
```python
def input_birthday():
while True:
try:
birthday = input("请输入您的生日(格式:月/日/年),例如12/31/1990:")
return format_birthday(birthday)
except ValueError:
print("输入格式错误,请重新输入。")
def format_birthday(birth_date_str):
month, day, year = birth_date_str.split('/')
# 检查月份和日期是否在有效范围内
if not (1 <= int(month) <= 12 and 1 <= int(day) <= 31):
raise ValueError("月份和日期超出范围,请输入1-31之间的数字.")
# 检查年份是否在有效范围内
if not (1000 <= int(year) <= 2099): # 更通用的做法是接受四位数年份
raise ValueError("年份超出范围,请输入公元后的四位数字.")
return f"{year}{month.zfill(2)}{day.zfill(2)}"
```
2. 存储日期:接着,你可以选择存储这个格式化的生日到文件、数据库或内存中,以便后续的提醒。
```python
def store_birthday(birth_date):
with open('birthdays.txt', 'a') as file:
file.write(f"{birth_date}\n")
# 调用函数并将结果存储
store_birthday(input_birthday())
```
3. 提醒功能:为了实现提醒,你可以设置定时任务或者在特定日期临近时检查存储的生日信息。这通常涉及到日历操作和比较当前日期。
```python
from datetime import datetime
def check_and_remind():
stored_birthdays = read_from_file() # 从文件读取存储的生日
today = datetime.today()
for stored_date in stored_birthdays:
stored_year, stored_month, stored_day = map(int, stored_date.split('/'))
reminder_date = datetime(stored_year, stored_month, stored_day)
if reminder_date - today < timedelta(days=7): # 设置7天内的提醒
print(f"提醒:今天是{stored_date}的生日!")
# 定期运行提醒检查
schedule.every().day.at("08:00").do(check_and_remind) # 每天早上8点运行
while True:
schedule.run_pending()
time.sleep(1)
```
阅读全文
相关推荐
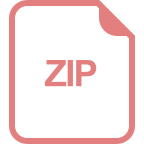
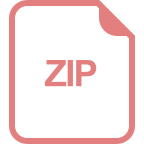
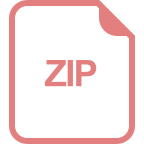

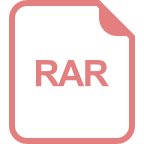













