7自由度机械臂轨迹规划代码
时间: 2023-10-25 15:10:12 浏览: 148
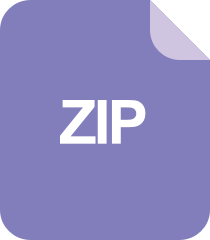
Matlab毕业设计—四自由度机械臂的轨迹规划研究.zip

以下是一个简单的7自由度机械臂轨迹规划代码示例,基于Python语言和ROS框架:
```python
import rospy
import math
import numpy as np
from geometry_msgs.msg import Pose
from sensor_msgs.msg import JointState
from moveit_msgs.msg import RobotTrajectory
from trajectory_msgs.msg import JointTrajectoryPoint
from moveit_msgs.srv import GetPositionIK, GetPositionIKRequest
class TrajectoryPlanner:
def __init__(self):
self.joint_names = ['joint_1', 'joint_2', 'joint_3', 'joint_4', 'joint_5', 'joint_6', 'joint_7']
self.current_joints = None
self.planning_time = 10.0
self.step_size = 0.01
self.jump_threshold = 0
self.ik_service = rospy.ServiceProxy('/compute_ik', GetPositionIK)
self.pub_traj = rospy.Publisher('/joint_trajectory', RobotTrajectory, queue_size=10)
def plan_path(self, start_pose, end_pose):
start_joints = self.compute_joints(start_pose)
end_joints = self.compute_joints(end_pose)
waypoints = self.interpolate(start_joints, end_joints)
trajectory = self.create_trajectory(waypoints)
self.pub_traj.publish(trajectory)
def compute_joints(self, pose):
ik_request = GetPositionIKRequest()
ik_request.ik_request.group_name = 'arm'
ik_request.ik_request.ik_seed_state.joint_state.name = self.joint_names
ik_request.ik_request.ik_seed_state.joint_state.position = self.current_joints
ik_request.ik_request.pose_stamped = pose
ik_response = self.ik_service(ik_request)
return ik_response.solution.joint_state.position
def interpolate(self, start_joints, end_joints):
waypoints = []
for t in np.arange(0.0, 1.0, self.step_size):
joints = []
for i in range(len(start_joints)):
s = start_joints[i]
e = end_joints[i]
if abs(e - s) <= math.pi:
j = s + t * (e - s)
else:
if e > s:
e -= 2 * math.pi
else:
e += 2 * math.pi
j = s + t * (e - s)
if j < -math.pi:
j += 2 * math.pi
elif j > math.pi:
j -= 2 * math.pi
joints.append(j)
waypoints.append(joints)
waypoints.append(end_joints)
return waypoints
def create_trajectory(self, waypoints):
trajectory = RobotTrajectory()
trajectory.joint_trajectory.joint_names = self.joint_names
for waypoint in waypoints:
point = JointTrajectoryPoint()
point.positions = waypoint
point.velocities = [0] * len(waypoints[0])
point.time_from_start = rospy.Duration(self.planning_time / len(waypoints))
trajectory.joint_trajectory.points.append(point)
return trajectory
def joint_state_callback(self, msg):
self.current_joints = msg.position
if __name__ == '__main__':
rospy.init_node('trajectory_planner')
planner = TrajectoryPlanner()
rospy.Subscriber('/joint_states', JointState, planner.joint_state_callback)
start_pose = Pose()
start_pose.position.x = 0.5
start_pose.position.y = 0.0
start_pose.position.z = 0.5
start_pose.orientation.x = 0.0
start_pose.orientation.y = 0.707
start_pose.orientation.z = 0.0
start_pose.orientation.w = 0.707
end_pose = Pose()
end_pose.position.x = 0.7
end_pose.position.y = 0.2
end_pose.position.z = 0.3
end_pose.orientation.x = 0.0
end_pose.orientation.y = 0.0
end_pose.orientation.z = 0.0
end_pose.orientation.w = 1.0
planner.plan_path(start_pose, end_pose)
rospy.spin()
```
这是一个简单的轨迹规划器,可以连接到机械臂的ROS节点上,通过输入起始和结束位姿来规划机械臂的运动轨迹。它使用ROS提供的MoveIt库来进行逆运动学求解和轨迹规划。在实际应用中,还需要考虑机械臂的动力学和碰撞检测等因素。
阅读全文
相关推荐
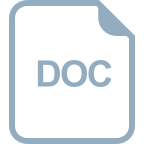
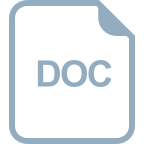


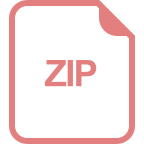
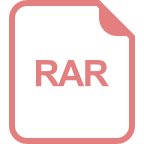
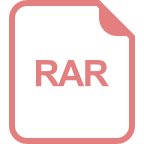
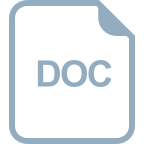
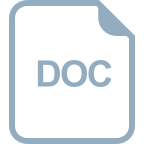
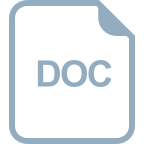
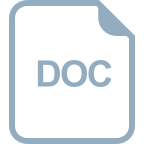
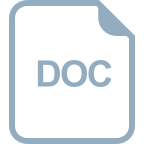
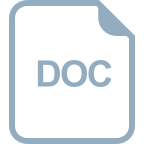
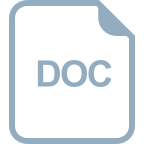
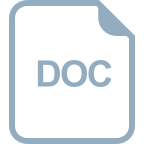

