用c语言设计一个基于DOS菜单的应用程序。内容如下: 1. 无向图的基本操作及应用 ① 创建无向图的邻接矩阵 ② 创建无向图的邻接表 ③ 无向图的深度优先遍历 ④ 无向图的广度优先遍历
时间: 2024-05-12 21:15:38 浏览: 97
以下是基于DOS菜单的无向图应用程序的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#define MAX_VERTICES 20
int graph[MAX_VERTICES][MAX_VERTICES]; //邻接矩阵
int visited[MAX_VERTICES]; //标记访问过的节点
int queue[MAX_VERTICES]; //队列
int front = 0, rear = 0; //队列的前后指针
typedef struct node {
int vertex; //节点编号
struct node *next; //指向下一个节点的指针
} Node;
Node *adj_list[MAX_VERTICES]; //邻接表
void create_graph_matrix(int vertices) {
int i, j;
for (i = 0; i < vertices; i++) {
for (j = 0; j < vertices; j++) {
graph[i][j] = 0; //初始化邻接矩阵
}
}
}
void create_graph_list(int vertices) {
int i;
for (i = 0; i < vertices; i++) {
adj_list[i] = NULL; //初始化邻接表
}
}
void add_edge_matrix(int start_vertex, int end_vertex) {
graph[start_vertex][end_vertex] = 1;
graph[end_vertex][start_vertex] = 1; //因为是无向图,所以需要两次添加
}
void add_edge_list(int start_vertex, int end_vertex) {
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->vertex = end_vertex;
new_node->next = adj_list[start_vertex];
adj_list[start_vertex] = new_node;
}
void dfs_matrix(int vertex, int vertices) {
int i;
visited[vertex] = 1;
printf("%d ", vertex);
for (i = 0; i < vertices; i++) {
if (graph[vertex][i] && !visited[i]) { //如果节点相邻且未被访问
dfs_matrix(i, vertices); //递归访问
}
}
}
void dfs_list(int vertex) {
Node *p = adj_list[vertex];
visited[vertex] = 1;
printf("%d ", vertex);
while (p != NULL) {
if (!visited[p->vertex]) { //如果节点未被访问
dfs_list(p->vertex); //递归访问
}
p = p->next;
}
}
void bfs_matrix(int vertex, int vertices) {
int i;
visited[vertex] = 1;
printf("%d ", vertex);
queue[rear++] = vertex; //将节点放入队列
while (front != rear) { //当队列不为空时
int current = queue[front++];
for (i = 0; i < vertices; i++) {
if (graph[current][i] && !visited[i]) { //如果节点相邻且未被访问
visited[i] = 1;
printf("%d ", i);
queue[rear++] = i; //将节点放入队列
}
}
}
}
void bfs_list(int vertex) {
Node *p;
visited[vertex] = 1;
printf("%d ", vertex);
queue[rear++] = vertex; //将节点放入队列
while (front != rear) { //当队列不为空时
int current = queue[front++];
p = adj_list[current];
while (p != NULL) {
if (!visited[p->vertex]) { //如果节点未被访问
visited[p->vertex] = 1;
printf("%d ", p->vertex);
queue[rear++] = p->vertex; //将节点放入队列
}
p = p->next;
}
}
}
void print_menu() {
printf("\n1. 创建无向图的邻接矩阵\n");
printf("2. 创建无向图的邻接表\n");
printf("3. 无向图的深度优先遍历 (邻接矩阵)\n");
printf("4. 无向图的深度优先遍历 (邻接表)\n");
printf("5. 无向图的广度优先遍历 (邻接矩阵)\n");
printf("6. 无向图的广度优先遍历 (邻接表)\n");
printf("7. 退出程序\n");
}
int main() {
int choice, vertices, edges, i, start_vertex, end_vertex;
while (1) {
print_menu();
printf("请选择: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入节点数: ");
scanf("%d", &vertices);
printf("请输入边数: ");
scanf("%d", &edges);
create_graph_matrix(vertices);
for (i = 0; i < edges; i++) {
printf("请输入第 %d 条边的起始节点和结束节点: ", i + 1);
scanf("%d%d", &start_vertex, &end_vertex);
add_edge_matrix(start_vertex, end_vertex);
}
break;
case 2:
printf("请输入节点数: ");
scanf("%d", &vertices);
printf("请输入边数: ");
scanf("%d", &edges);
create_graph_list(vertices);
for (i = 0; i < edges; i++) {
printf("请输入第 %d 条边的起始节点和结束节点: ", i + 1);
scanf("%d%d", &start_vertex, &end_vertex);
add_edge_list(start_vertex, end_vertex);
}
break;
case 3:
printf("请输入起始节点: ");
scanf("%d", &start_vertex);
for (i = 0; i < vertices; i++) {
visited[i] = 0; //初始化访问标记
}
dfs_matrix(start_vertex, vertices);
break;
case 4:
printf("请输入起始节点: ");
scanf("%d", &start_vertex);
for (i = 0; i < vertices; i++) {
visited[i] = 0; //初始化访问标记
}
dfs_list(start_vertex);
break;
case 5:
printf("请输入起始节点: ");
scanf("%d", &start_vertex);
for (i = 0; i < vertices; i++) {
visited[i] = 0; //初始化访问标记
}
bfs_matrix(start_vertex, vertices);
break;
case 6:
printf("请输入起始节点: ");
scanf("%d", &start_vertex);
for (i = 0; i < vertices; i++) {
visited[i] = 0; //初始化访问标记
}
bfs_list(start_vertex);
break;
case 7:
exit(0); //退出程序
default:
printf("无效的选项,请重试\n");
break;
}
}
return 0;
}
```
注意:本程序只适用于DOS环境,如果您在Windows或Linux等其他操作系统上运行,可能会出现兼容性问题。
阅读全文
相关推荐
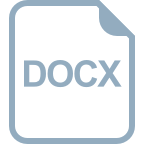
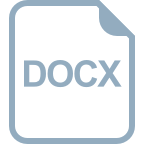
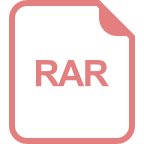















