使用C语言编写一个应用栈判断英语单词回文词以及其个数
时间: 2024-10-13 18:09:54 浏览: 32
在C语言中,我们可以创建一个栈来辅助检查一个字符串是否是回文。首先,需要定义一个栈结构体,并实现基本的入栈(push)、出栈(pop)操作。然后,我们遍历输入的英文单词,将非空格字符依次压入栈中,同时记录每个单词的长度。接下来,从头到尾再次遍历单词,每次取出栈顶元素并与当前字符比较,如果相等则继续,如果不等则该单词不是回文。最后统计回文词的数量。
以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORDS 100
#define MAX_LENGTH 50
typedef struct {
char word[MAX_LENGTH];
int count;
} WordInfo;
// Stack structure with capacity
struct Stack {
WordInfo words[MAX_WORDS];
int top;
};
void push(Stack* stack, const char* word) {
if (stack->top == MAX_WORDS - 1) {
printf("Stack overflow, cannot add more words.\n");
return;
}
strcpy(stack->words[stack->top].word, word);
stack->words[stack->top++].count = 1;
}
WordInfo pop(Stack* stack) {
if (stack->top == 0) {
printf("Stack underflow, no more words to remove.\n");
return (WordInfo){NULL, 0};
}
WordInfo info = stack->words[--stack->top];
memset(info.word, 0, sizeof(info.word));
return info;
}
int isPalindrome(const char* word) {
int start = 0, end = strlen(word) - 1;
while (start < end && word[start] == word[end]) {
start++;
end--;
}
return start >= end;
}
int main() {
Stack stack;
stack.top = 0;
// Input example
const char* input = "Able was I ere I saw Elba.";
char* token;
token = strtok(input, " ");
while (token != NULL) {
push(&stack, token);
if (isPalindrome(token)) {
printf("%s is a palindrome.\n", token);
}
token = strtok(NULL, " ");
}
WordInfo current;
while (!stack.isEmpty()) {
current = pop(&stack);
if (current.count > 1) {
printf("%s appears %d times and is a palindrome.\n", current.word, current.count);
}
}
return 0;
}
```
这个程序会统计输入字符串中的回文词及其出现次数。注意,这里假设了单词之间由空格分隔。如果单词包含标点符号或特殊字符,你需要先处理掉这些字符再进行分析。
阅读全文
相关推荐
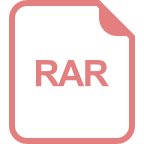
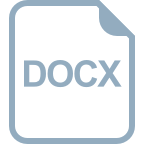

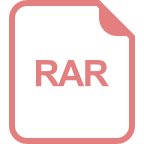
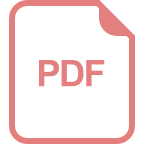
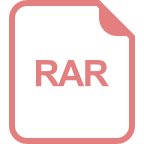
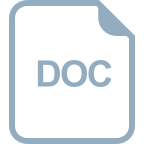





