python 日程管理程序具有添加日程、查询日程(由关键字日期/时间/标题/内容查询)、删除日程、显示日程列表、编辑日程和退出程序的功能
时间: 2024-05-04 21:22:10 浏览: 131
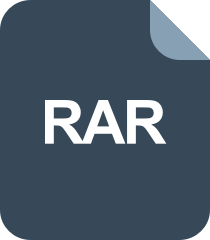
日程管理软件

好的,这个程序需要用到一些库,比如datetime、os等。我们可以先定义一个主函数,然后再逐个实现这些功能。以下是一个简单的日程管理程序:
```python
import datetime
import os
def main():
while True:
print('请选择操作:')
print('1. 添加日程')
print('2. 查询日程')
print('3. 删除日程')
print('4. 显示日程列表')
print('5. 编辑日程')
print('6. 退出程序')
choice = input('请输入操作编号:')
if choice == '1':
add_schedule()
elif choice == '2':
query_schedule()
elif choice == '3':
delete_schedule()
elif choice == '4':
show_schedule_list()
elif choice == '5':
edit_schedule()
elif choice == '6':
print('程序已退出。')
break
else:
print('输入有误,请重新输入。')
def add_schedule():
print('请输入日程信息:')
title = input('标题:')
content = input('内容:')
date_str = input('日期(格式为YYYY-MM-DD):')
time_str = input('时间(格式为HH:MM):')
datetime_str = date_str + ' ' + time_str
try:
schedule_time = datetime.datetime.strptime(datetime_str, '%Y-%m-%d %H:%M')
except ValueError:
print('日期或时间格式不正确,请重新输入。')
return
schedule = {'title': title, 'content': content, 'time': schedule_time}
with open('schedule.txt', 'a') as f:
f.write(str(schedule) + '\n')
print('日程添加成功。')
def query_schedule():
keyword = input('请输入查询关键字:')
with open('schedule.txt', 'r') as f:
schedules = f.readlines()
for schedule in schedules:
schedule = eval(schedule)
if keyword in schedule['title'] or keyword in schedule['content'] or keyword in str(schedule['time']):
print(schedule)
def delete_schedule():
keyword = input('请输入待删除日程的关键字:')
with open('schedule.txt', 'r') as f:
schedules = f.readlines()
new_schedules = []
for schedule in schedules:
schedule = eval(schedule)
if keyword not in schedule['title'] and keyword not in schedule['content'] and keyword not in str(schedule['time']):
new_schedules.append(schedule)
with open('schedule.txt', 'w') as f:
for schedule in new_schedules:
f.write(str(schedule) + '\n')
print('日程删除成功。')
def show_schedule_list():
with open('schedule.txt', 'r') as f:
schedules = f.readlines()
for schedule in schedules:
print(schedule)
def edit_schedule():
keyword = input('请输入待编辑日程的关键字:')
with open('schedule.txt', 'r') as f:
schedules = f.readlines()
for i, schedule in enumerate(schedules):
schedule = eval(schedule)
if keyword in schedule['title'] or keyword in schedule['content'] or keyword in str(schedule['time']):
print(schedule)
new_title = input('请输入新标题(如不修改请直接按回车):')
new_content = input('请输入新内容(如不修改请直接按回车):')
new_date_str = input('请输入新日期(格式为YYYY-MM-DD,如不修改请直接按回车):')
new_time_str = input('请输入新时间(格式为HH:MM,如不修改请直接按回车):')
if new_title:
schedule['title'] = new_title
if new_content:
schedule['content'] = new_content
if new_date_str and new_time_str:
new_datetime_str = new_date_str + ' ' + new_time_str
try:
new_schedule_time = datetime.datetime.strptime(new_datetime_str, '%Y-%m-%d %H:%M')
schedule['time'] = new_schedule_time
except ValueError:
print('日期或时间格式不正确,修改失败。')
schedules[i] = str(schedule) + '\n'
with open('schedule.txt', 'w') as f:
for schedule in schedules:
f.write(schedule)
print('日程编辑成功。')
if __name__ == '__main__':
main()
```
该程序将日程保存在名为`schedule.txt`的文件中,每行为一个字典对象表示一个日程。可以根据需要自行修改文件名和格式。同时,该程序还没有对输入进行合法性检查,如输入日期和时间的格式是否正确、输入的关键字是否存在等,建议在实际应用中添加相应的检查。
阅读全文
相关推荐










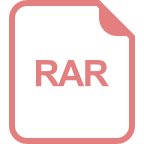