vue3强制刷新子组件
时间: 2023-11-15 20:02:36 浏览: 69
在Vue3中,强制刷新子组件的方法与Vue2略有不同。可以使用`markRaw`函数将数据标记为“原始”,从而避免响应式系统对其进行追踪,然后在子组件中使用`toRaw`函数将其转换为原始数据,从而避免子组件的响应式更新。具体实现方法如下:
1. 在父组件中,将需要强制刷新的数据使用`markRaw`函数标记为原始数据:
```
import { markRaw } from 'vue'
export default {
data() {
return {
rawData: markRaw({ /* your data */ })
}
}
}
```
2. 在子组件中,使用`toRaw`函数将原始数据转换为普通数据:
```
import { toRaw } from 'vue'
export default {
props: ['data'],
setup(props) {
const data = toRaw(props.data)
// ...
}
}
```
3. 在需要强制刷新子组件的时候,可以使用`$forceUpdate`方法强制重新渲染子组件:
```
this.$forceUpdate()
```
相关问题
vue 父组件强制刷新子组件
你可以通过两种方式强制刷新Vue子组件:
1. 使用 `key` 属性:在父组件中给子组件添加一个唯一的 `key` 属性,并在需要强制刷新子组件时,改变 `key` 的值。这将导致Vue重新渲染子组件,达到强制刷新的效果。
```html
<template>
<div>
<child-component :key="componentKey"></child-component>
<button @click="refreshChild">刷新子组件</button>
</div>
</template>
<script>
export default {
data() {
return {
componentKey: 0
};
},
methods: {
refreshChild() {
this.componentKey += 1;
}
}
};
</script>
```
2. 使用 `$forceUpdate` 方法:在父组件中,可以直接调用 `$forceUpdate` 方法来强制刷新子组件。这个方法会触发Vue重新渲染组件及其子组件。
```html
<template>
<div>
<child-component></child-component>
<button @click="refreshChild">刷新子组件</button>
</div>
</template>
<script>
export default {
methods: {
refreshChild() {
this.$forceUpdate();
}
}
};
</script>
```
请注意,`$forceUpdate` 方法会重新渲染整个组件及其子组件,而不仅仅是子组件本身。因此,它可能会有一些性能开销,所以建议在必要时使用。
vue中父组件强制刷新子组件
在Vue中,父组件可以通过修改子组件的属性或触发子组件的事件来更新子组件。但是,如果父组件想要强制刷新子组件,可以使用Vue的key特性。
Vue的key特性可以用于强制重新渲染组件。当一个组件的key发生改变时,Vue会销毁旧的组件并创建一个新的组件,从而实现强制刷新的效果。
例如,在父组件中,可以给子组件添加一个key,然后在需要刷新子组件的时候,通过修改子组件的key来强制刷新子组件。示例代码如下:
```html
<template>
<div>
<child-component :key="childKey"></child-component>
<button @click="refreshChild">刷新子组件</button>
</div>
</template>
<script>
export default {
data() {
return {
childKey: 0,
};
},
methods: {
refreshChild() {
this.childKey += 1;
},
},
};
</script>
```
在上面的代码中,父组件中的`childKey`属性被用作子组件的key。当点击“刷新子组件”按钮时,父组件会通过修改`childKey`的值来强制刷新子组件。
相关推荐
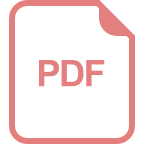












