numpy 字符串与 python 字符串互相转换
时间: 2023-11-29 17:04:12 浏览: 157
可以使用numpy的函数`numpy.char.encode`和`numpy.char.decode`来实现numpy字符串与python字符串的互相转换。具体步骤如下:
将python字符串转换为numpy字符串:
```python
import numpy as np
py_str = 'hello world'
np_str = np.char.encode(py_str, encoding='UTF-8')
print(np_str)
```
输出结果为:
```
[b'hello world']
```
将numpy字符串转换为python字符串:
```python
py_str = np.char.decode(np_str, encoding='UTF-8')
print(py_str)
```
输出结果为:
```
hello world
```
相关问题
将numpy数组字符串转为python字符串
你可以使用`numpy.array_str()`方法将Numpy数组转换为字符串,然后使用Python内置的`str()`函数将其转换为Python字符串。例如:
```python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
arr_str = np.array_str(arr)
py_str = str(arr_str)
print(py_str)
```
输出结果为:`'[1 2 3 4 5]'`
python numpy 字符串转时间
在Python中,NumPy库主要用于数值计算,但它本身并不直接支持字符串转时间的操作。如果你需要将字符串转换成时间,通常会结合`pandas`库或者是`datetime`模块来完成。`datetime`模块是标准库的一部分,专门用于处理日期和时间。
例如,你可以按照以下步骤将一个包含日期字符串的列转换为时间格式:
```python
from datetime import datetime
# 假设你有一个字符串列表
date_strings = ['2022-01-01', '2022-02-14']
# 使用strptime函数解析字符串
time_list = [datetime.strptime(date_string, '%Y-%m-%d') for date_string in date_strings]
print(time_list)
```
这里,`'%Y-%m-%d'`是一个格式化字符串,代表年-月-日的顺序。`strptime`函数根据这个格式从字符串中提取出对应的日期并创建`datetime`对象。
如果你想要在numpy数组上操作,可以先转换为`pandas`的数据结构,如Series,然后利用`pandas`的`to_datetime`函数:
```python
import pandas as pd
date_series = pd.Series(date_strings)
datetime_series = pd.to_datetime(date_series)
print(datetime_series)
```
阅读全文
相关推荐
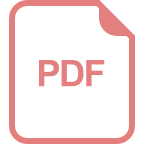
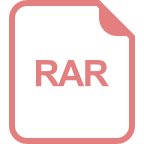
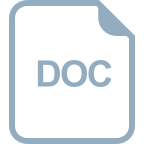









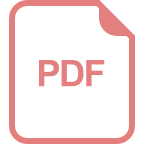

