numpy 判断字符串
时间: 2023-07-26 21:22:08 浏览: 105
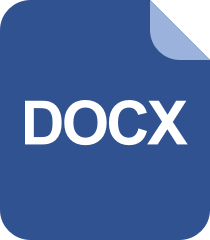
python.docx
NumPy没有专门用于字符串判断的函数,但可以使用字符串方法和NumPy的向量化操作来判断字符串。以下是一些示例:
1. 判断字符串是否以特定字符开头:
```python
import numpy as np
arr = np.array(['hello', 'world', 'numpy'])
result = np.char.startswith(arr, 'h')
print(result) # [ True False False]
```
2. 判断字符串是否包含特定字符:
```python
arr = np.array(['hello', 'world', 'numpy'])
result = np.char.find(arr, 'l')
print(result) # [ 2 -1 1]
```
3. 判断字符串是否为数字:
```python
arr = np.array(['123', 'world', 'numpy'])
result = np.char.isdigit(arr)
print(result) # [ True False False]
```
这些函数都可以用于NumPy数组中的所有元素,而不需要使用循环来遍历数组。
阅读全文
相关推荐
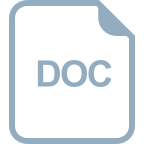
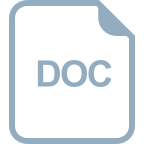


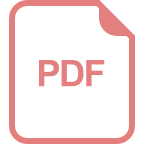
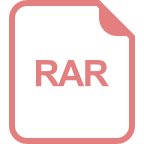
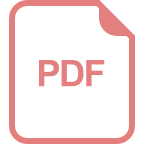
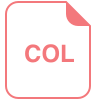
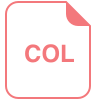
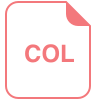
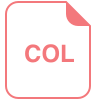
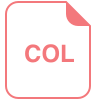
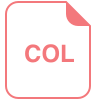
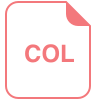
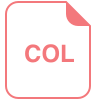
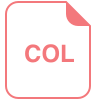

