c++编程 定义时钟类Clock(数据成员为时、分、秒),设置成员函数ShowTime实现对时间的输出。实现对时钟类对象的自增自减运算(包括前置和后置)。程序中定义Clock类对象t1和t2,输入t1的值。整型变量n的值为如下设定: (1)输入n的值为1,执行 t2=t1++; t1.ShowTime(); t2.ShowTime(); (2)输入n的值为2,执行 t2=t1--; t1.ShowTime(); t2.ShowTime(); (3)输入n的值为3,执行 t2=++t1; t1.ShowTime(); t2.ShowTime(); (4)输入n的值为4,执行 t2=--t1; t1.ShowTime(); t2.ShowTime();
时间: 2024-03-14 20:46:55 浏览: 44
以下是Clock类的实现代码:
```c++
#include <iostream>
using namespace std;
class Clock {
private:
int hour;
int minute;
int second;
public:
Clock(int h = 0, int m = 0, int s = 0) {
hour = h;
minute = m;
second = s;
}
void ShowTime() {
cout << hour << ":" << minute << ":" << second << endl;
}
Clock operator++() { // 前置自增
second++;
if (second >= 60) {
second = 0;
minute++;
}
if (minute >= 60) {
minute = 0;
hour++;
}
if (hour >= 24) {
hour = 0;
}
return *this;
}
Clock operator++(int) { // 后置自增
Clock old = *this;
++(*this);
return old;
}
Clock operator--() { // 前置自减
second--;
if (second < 0) {
second = 59;
minute--;
}
if (minute < 0) {
minute = 59;
hour--;
}
if (hour < 0) {
hour = 23;
}
return *this;
}
Clock operator--(int) { // 后置自减
Clock old = *this;
--(*this);
return old;
}
};
```
下面是按照题目要求执行的代码:
```c++
int main() {
int h, m, s, n;
cout << "请输入t1的值(时 分 秒):";
cin >> h >> m >> s;
Clock t1(h, m, s), t2;
cout << "请输入n的值:";
cin >> n;
switch (n) {
case 1:
t2 = t1++;
t1.ShowTime();
t2.ShowTime();
break;
case 2:
t2 = t1--;
t1.ShowTime();
t2.ShowTime();
break;
case 3:
t2 = ++t1;
t1.ShowTime();
t2.ShowTime();
break;
case 4:
t2 = --t1;
t1.ShowTime();
t2.ShowTime();
break;
default:
cout << "无效的n值" << endl;
}
return 0;
}
```
注意,前置自增和前置自减都是直接修改对象的值并返回修改后的对象,而后置自增和后置自减则是先返回对象的旧值再修改对象的值。这里的实现中,我们通过传入一个 int 类型的参数来区分前置和后置自增自减运算符的重载函数。
相关推荐
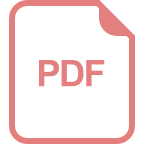
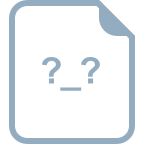
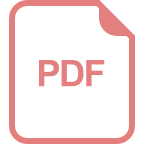














