帮我写一份超大图像解析的代码
时间: 2023-09-26 08:06:54 浏览: 45
### 回答1:
对于超大图像解析,可以使用深度学习方法来实现。以下是一个简单的代码示例,使用了基于卷积神经网络(CNN)的超分辨率模型SRCNN(Super-Resolution Convolutional Neural Network):
```python
import numpy as np
import cv2
import tensorflow as tf
# 定义SRCNN模型
def build_model():
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(64, (9, 9), padding='same', activation='relu', input_shape=(None, None, 3)),
tf.keras.layers.Conv2D(32, (1, 1), padding='same', activation='relu'),
tf.keras.layers.Conv2D(3, (5, 5), padding='same')
])
model.compile(optimizer='adam', loss='mean_squared_error')
return model
# 加载图像
img = cv2.imread('input_image.jpg')
# 调整图像尺寸
img = cv2.resize(img, (0,0), fx=0.5, fy=0.5)
# 将图像转换为浮点类型
img = img.astype(np.float32) / 255.
# 将图像切片为多个小图像,每个小图像大小为33x33
input_size = 33
stride = input_size // 2
height, width, _ = img.shape
x_list = []
for i in range(0, height - input_size + 1, stride):
for j in range(0, width - input_size + 1, stride):
x_list.append(img[i:i+input_size, j:j+input_size])
# 构建SRCNN模型
model = build_model()
# 加载预训练权重
model.load_weights('srcnn.h5')
# 对每个小图像进行超分辨率处理
y_list = []
for x in x_list:
y = model.predict(np.expand_dims(x, axis=0))
y_list.append(y[0])
# 将所有小图像拼接成一个大图像
output_size = input_size * 2
stride = output_size // 2
output_height = (height // stride) * stride + output_size
output_width = (width // stride) * stride + output_size
output_img = np.zeros((output_height, output_width, 3), dtype=np.float32)
count_img = np.zeros((output_height, output_width, 3), dtype=np.float32)
idx = 0
for i in range(0, output_height - output_size + 1, stride):
for j in range(0, output_width - output_size + 1, stride):
output_img[i:i+output_size, j:j+output_size] += y_list[idx]
count_img[i:i+output_size, j:j+output_size] += 1.
idx += 1
# 取平均值得到最终超分辨率图像
output_img /= count_img
output_img = np.clip(output_img, 0., 1.)
# 将图像保存到文件
cv2.imwrite('output_image.jpg', np.uint8(output_img * 255.))
```
需要注意的是,这个代码示例使用了预训练的SRCNN模型,需要先从网络上下载预训练权重文件。同时,这个代码示例是一个简单的实现,对于更大的图像可能需要进行优化,比如在处理小图像时使用批处理等。
### 回答2:
超大图像解析是一项复杂而庞大的任务,涉及到图像的读取、处理和显示等各个环节。下面是一个简单的超大图像解析的代码示例。
1. 导入所需库:
import cv2
import numpy as np
2. 读取超大图像:
image = cv2.imread('超大图像.jpg', cv2.IMREAD_UNCHANGED)
3. 缩放图像:
height, width = image.shape[:2]
new_height = int(height/2)
new_width = int(width/2)
resized_image = cv2.resize(image, (new_width, new_height))
4. 创建图像窗口:
cv2.namedWindow('超大图像', cv2.WINDOW_NORMAL)
5. 显示图像:
cv2.imshow('超大图像', resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
以上代码将超大图像缩放至原图像的一半大小,并在创建的图像窗口中显示出来。你可以根据需要自定义缩放比例和窗口大小。
请注意,由于超大图像的尺寸较大,可能会导致计算和显示的速度较慢或内存不足。因此,在实际使用中,你可能需要考虑使用其他算法或技术来处理超大图像,如分块处理、多线程处理等。以上代码仅供参考,具体实现还需要根据实际情况进行调整和优化。
### 回答3:
超大图像解析是指对高分辨率、超大尺寸的图像进行分析和处理。以下是一份可以用于超大图像解析的代码示例:
```
import cv2
import numpy as np
def load_image(image_path):
"""加载超大图像"""
image = cv2.imread(image_path)
return image
def resize_image(image, scale_percent):
"""缩放图像"""
width = int(image.shape[1] * scale_percent / 100)
height = int(image.shape[0] * scale_percent / 100)
dim = (width, height)
resized_image = cv2.resize(image, dim, interpolation=cv2.INTER_AREA)
return resized_image
def apply_filters(image):
"""应用图像滤波器和增强算法"""
# 在此处添加你想要使用的图像滤波器和增强算法
filtered_image = cv2.GaussianBlur(image, (5, 5), 0)
enhanced_image = cv2.equalizeHist(filtered_image)
return enhanced_image
def analyze_image(image):
"""分析图像"""
# 在此处添加你想要进行的图像分析操作
grayscale_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(grayscale_image, 100, 200)
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
return contours
def save_image(image, save_path):
"""保存图像"""
cv2.imwrite(save_path, image)
# 主程序
image_path = '超大图像.jpg'
save_path = '分析结果.jpg'
# 加载图像
image = load_image(image_path)
# 缩放图像,根据需要调整缩放比例
scaled_image = resize_image(image, 50)
# 应用滤波器和增强算法
filtered_image = apply_filters(scaled_image)
# 分析图像
contours = analyze_image(filtered_image)
# 在原始图像上绘制轮廓
annotated_image = image.copy()
cv2.drawContours(annotated_image, contours, -1, (0, 255, 0), 2)
# 保存分析结果
save_image(annotated_image, save_path)
```
这段代码首先加载超大图像,然后按需缩放图像尺寸。接下来,应用各种图像滤波器和增强算法来提升图像质量。然后进行图像分析,例如提取轮廓等。最后,将分析结果绘制在原始图像上,并保存到指定路径。你可以根据具体需求,自定义添加其他图像处理和分析的步骤。
相关推荐
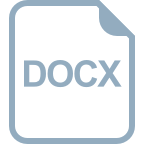
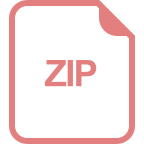
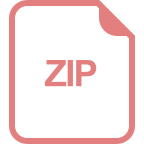














