帮我解释下用hbuilder开发工具使用vue语言如何实现手机壁纸小程序点击不同分类显示不同类型图片,需要详细代码并注释解释每步代码的含义,我是零基础没有接触过it行业,通俗解释小程序开发到上线的过程,包括使用uinicloud数据库,云函数相关配置的知识点,需要学习哪些知识点,云函数怎么开发,数据库如何存储图片并分类
时间: 2024-03-01 08:55:41 浏览: 23
好的,我会尽力详细地解释。首先,我们需要了解几个概念。
1. HBuilder开发工具:HBuilder是一款集成了多个开发工具的IDE,可以用于开发多种移动端应用程序,包括小程序。
2. Vue语言:Vue是一种流行的JavaScript框架,用于构建Web应用程序。在小程序开发中,Vue可以用来构建组件。
3. 手机壁纸小程序:这是一个小程序,用于提供不同类型的手机壁纸。用户可以选择不同的分类来查看不同类型的壁纸。
现在让我们来看看如何使用HBuilder开发工具和Vue语言来实现这个小程序的功能。
1. 创建页面
首先,我们需要在HBuilder中创建一个页面。在页面中,我们可以使用Vue语言来构建组件。比如,我们可以使用vue-router来实现不同分类的切换。
创建一个index.vue文件,代码如下:
```html
<template>
<div>
<van-tabbar v-model="active" fixed>
<van-tabbar-item icon="home-o">首页</van-tabbar-item>
<van-tabbar-item icon="search">分类</van-tabbar-item>
<van-tabbar-item icon="friends-o">我的</van-tabbar-item>
</van-tabbar>
<van-image :src="image"></van-image>
</div>
</template>
<script>
export default {
data() {
return {
active: 0,
image: ''
};
},
methods: {
getImage() {
// 获取图片数据的逻辑
}
},
mounted() {
this.getImage();
}
};
</script>
```
2. 使用组件
在页面中,我们可以使用不同的组件来展示不同的内容。比如,我们可以使用vant组件库中的图片组件来展示图片。我们还可以使用自定义组件来创建一些特定的功能,比如分类切换按钮组件。
修改index.vue文件,代码如下:
```html
<template>
<div>
<van-tabbar v-model="active" fixed>
<van-tabbar-item icon="home-o">首页</van-tabbar-item>
<van-tabbar-item icon="search">分类</van-tabbar-item>
<van-tabbar-item icon="friends-o">我的</van-tabbar-item>
</van-tabbar>
<van-image :src="image"></van-image>
<div v-if="active === 1">
<van-button @click="setCategory(1)">分类1</van-button>
<van-button @click="setCategory(2)">分类2</van-button>
<van-button @click="setCategory(3)">分类3</van-button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
active: 0,
image: '',
category: 1
};
},
methods: {
getImage() {
// 获取图片数据的逻辑
},
setCategory(category) {
this.category = category;
// 获取分类数据的逻辑
}
},
mounted() {
this.getImage();
}
};
</script>
```
3. 使用API
在小程序中,我们可以使用API来访问后台数据。在这个小程序中,我们可以使用uinicloud数据库来存储图片数据。我们还可以使用云函数来处理一些业务逻辑,比如获取图片数据、分类筛选等等。
修改index.vue文件,代码如下:
```html
<template>
<div>
<van-tabbar v-model="active" fixed>
<van-tabbar-item icon="home-o">首页</van-tabbar-item>
<van-tabbar-item icon="search">分类</van-tabbar-item>
<van-tabbar-item icon="friends-o">我的</van-tabbar-item>
</van-tabbar>
<van-image :src="image"></van-image>
<div v-if="active === 1">
<van-button @click="setCategory(1)">分类1</van-button>
<van-button @click="setCategory(2)">分类2</van-button>
<van-button @click="setCategory(3)">分类3</van-button>
<van-image v-for="(item, index) in images" :key="index" :src="item"></van-image>
</div>
</div>
</template>
<script>
export default {
data() {
return {
active: 0,
image: '',
category: 1,
images: []
};
},
methods: {
getImage() {
const db = uniCloud.database();
db.collection('images').where({
category: this.category
}).get().then(res => {
this.images = res.data.map(item => item.src);
});
},
setCategory(category) {
this.category = category;
this.getImage();
}
},
mounted() {
this.getImage();
}
};
</script>
```
4. 部署上线
最后,我们需要将小程序部署到线上环境中。在这个过程中,我们需要了解一些基本的知识点,比如小程序的AppID、域名配置、代码上传等等。
需要学习的知识点包括:Vue框架、vue-router、vant组件库、uinicloud数据库、云函数、小程序开发基础知识(AppID、域名配置、代码上传等等)。
接下来我们来解释一下代码的含义:
1. 在template标签中,我们使用了vant组件库中的van-tabbar、van-tabbar-item、van-image、van-button组件。van-tabbar用于实现底部导航栏,van-image用于展示图片,van-button用于分类切换按钮。
2. 在script标签中,我们定义了四个变量:active、image、category、images。active表示当前所在的tabbar项,image表示当前展示的图片,category表示当前选择的分类,images表示当前分类下的所有图片。
3. 在methods中,我们定义了两个方法:getImage和setCategory。getImage方法用于获取当前分类下的所有图片,setCategory方法用于切换分类。
4. 在mounted生命周期函数中,我们调用了getImage方法,以便在页面加载时获取默认分类下的所有图片。
在小程序开发到上线的过程中,我们需要了解一些基础知识,包括小程序的AppID、域名配置、代码上传等等。在使用uinicloud数据库和云函数时,我们需要学习相关的知识点,包括如何创建数据库、如何编写云函数等等。
关于数据库如何存储图片并分类,我们可以在数据库中创建一个images表,每个记录包含两个字段:src和category。src表示图片地址,category表示图片所属的分类。我们可以在云函数中通过调用数据库API来实现图片的存储和分类。
相关推荐
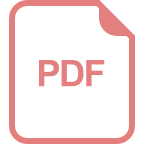
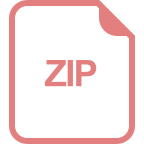














