computed: { enable() { const columns = this.$refs.table.columns; const enableColumns = columns.filter(column => column.id === 'enable'); return enableColumns; }, },这段代码有错误吗
时间: 2024-03-13 18:43:47 浏览: 64
这段代码的语法没有错误,但是可能会存在一些逻辑上的问题。
在这段代码中,computed属性`enable`的值是由对`$refs.table`的引用进行计算得出的。在计算过程中,会从`$refs.table.columns`数组中筛选出所有`id`属性值为`'enable'`的元素,返回一个新的数组。最后,这个新数组将作为`enable`属性的值返回。
但是,这个计算属性返回的是一个数组,而不是一个布尔值。如果您希望返回一个布尔值,您可以将`enableColumns`数组的长度作为计算属性的值返回,例如:
```
computed: {
enable() {
const columns = this.$refs.table.columns;
const enableColumns = columns.filter(column => column.id === 'enable');
return enableColumns.length > 0;
},
},
```
这样计算属性`enable`的值就会是一个布尔值,表示是否存在`id`属性值为`'enable'`的列。
相关问题
<el-table border :data="infoDataList" max-height="300" :row-class-name="tableRowClassName">不使用插件,如何使只加载可视部分
好的,你的问题是如何不使用插件实现只加载可视部分的`<el-table>`表格。在Vue.js中,你可以使用`v-show`指令和计算属性来实现这一功能。以下是实现方法:
1. 在你的Vue.js组件中,定义一个计算属性来计算当前可见的行数。例如:
```javascript
computed: {
visibleRowCount() {
const table = this.$refs['my-table'].$el.querySelector('.el-table__body-wrapper');
const rowHeight = 40; // 每行的高度
const visibleHeight = table.clientHeight; // 可见区域的高度
return Math.ceil(visibleHeight / rowHeight);
}
}
```
这个计算属性会计算可见区域内的行数,假设每行高度为40像素。
2. 使用`v-show`指令来控制每行的可见性。例如:
```html
<template>
<el-table ref="my-table" border max-height="300" :row-class-name="tableRowClassName">
<el-table-column
v-for="(column, index) in columns"
:key="index"
:prop="column.prop"
:label="column.label"
>
</el-table-column>
<el-table-column
fixed="right"
label="操作"
width="100"
>
<template slot-scope="scope">
<el-button type="text" size="small">编辑</el-button>
<el-button type="text" size="small">删除</el-button>
</template>
</el-table-column>
<el-table-body>
<el-table-row
v-for="(row, index) in infoDataList"
:key="index"
v-show="index >= startIndex && index < endIndex"
>
<el-table-column
v-for="(column, index) in columns"
:key="index"
:prop="column.prop"
>
{{ row[column.prop] }}
</el-table-column>
</el-table-row>
</el-table-body>
</el-table>
</template>
```
在上面的代码中,我们使用`v-show`指令来控制每行的可见性。`startIndex`和`endIndex`是计算属性`visibleRowCount`计算出的可见行的起始和结束索引。只有在这个范围内的行才会被渲染和显示。
通过这种方式,`<el-table>`表格将只渲染可见的部分,同时保持高性能。希望这个方法对你有所帮助!
el-table虚拟列表实现代码
以下是el-table虚拟列表的实现代码:
```javascript
<template>
<el-table
ref="table"
:data="tableData"
:row-key="row => row.id"
:height="tableHeight"
:row-height="rowHeight"
:header-row-height="headerRowHeight"
:v-loading="loading"
:default-sort="defaultSort"
@sort-change="handleSortChange"
@row-click="handleRowClick"
@row-contextmenu="handleRowContextMenu"
>
<el-table-column
v-for="column in columns"
:key="column.prop"
:label="column.label"
:prop="column.prop"
:min-width="column.minWidth"
:resizable="column.resizable"
:formatter="column.formatter"
:sortable="column.sortable"
:sort-method="column.sortMethod"
:align="column.align"
:header-align="column.headerAlign"
:show-overflow-tooltip="column.showOverflowTooltip"
:fixed="column.fixed"
/>
</el-table>
</template>
<script>
import { debounce } from 'lodash'
export default {
name: 'VirtualTable',
props: {
columns: {
type: Array,
required: true
},
data: {
type: Array,
required: true
},
total: {
type: Number,
required: true
},
loading: {
type: Boolean,
default: false
},
height: {
type: Number,
required: true
},
rowHeight: {
type: Number,
default: 40
},
headerRowHeight: {
type: Number,
default: 40
},
defaultSort: {
type: Object
},
fetch: {
type: Function,
required: true
}
},
data() {
return {
tableData: [],
scrollTop: 0,
startRow: 0,
endRow: 0,
visibleRowCount: 0,
requestParams: {},
sort: null
}
},
computed: {
tableHeight() {
return this.height - this.headerRowHeight
},
visibleRowStartIndex() {
return Math.floor(this.scrollTop / this.rowHeight)
},
visibleRowEndIndex() {
return Math.floor((this.scrollTop + this.tableHeight) / this.rowHeight)
},
isAllDataLoaded() {
return this.endRow === this.total - 1
}
},
watch: {
data() {
this.loadData()
}
},
mounted() {
this.loadData()
this.$refs.table.bodyWrapper.addEventListener('scroll', debounce(this.handleScroll, 50))
},
methods: {
async loadData() {
this.tableData = []
this.startRow = 0
this.endRow = 0
this.visibleRowCount = 0
if (this.data.length > 0) {
await this.loadRows()
this.$refs.table.doLayout()
}
},
async loadRows() {
this.requestParams = {
start: this.startRow,
end: this.endRow,
sort: this.sort
}
const result = await this.fetch(this.requestParams)
this.tableData = this.tableData.concat(result.data)
this.startRow = this.endRow + 1
this.endRow = this.startRow + result.data.length - 1
this.visibleRowCount = this.tableData.length
},
async handleScroll() {
const { scrollTop } = this.$refs.table.bodyWrapper
const scrollDistance = Math.abs(scrollTop - this.scrollTop)
if (scrollDistance > this.rowHeight) {
this.scrollTop = scrollTop
await this.updateVisibleRows()
}
},
async updateVisibleRows() {
let shouldLoadMore = false
if (this.visibleRowCount === 0) {
shouldLoadMore = true
} else if (this.visibleRowStartIndex > this.endRow || this.visibleRowEndIndex < this.startRow) {
shouldLoadMore = true
}
if (shouldLoadMore && !this.isAllDataLoaded) {
await this.loadRows()
}
},
async handleSortChange(sort) {
this.sort = sort
await this.loadData()
},
async handleRowClick(row, column, event) {
this.$emit('row-click', row, column, event)
},
async handleRowContextMenu(row, column, event) {
this.$emit('row-contextmenu', row, column, event)
}
}
}
</script>
```
该组件的实现主要是通过监听表格的滚动事件来动态加载数据,从而实现虚拟列表。同时,还有一些其他的功能,如排序、点击行、右键菜单等,都已经在代码中实现。需要注意的是,这里使用了 lodash 库中的 debounce 函数来控制滚动事件的触发频率。
阅读全文
相关推荐
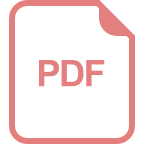
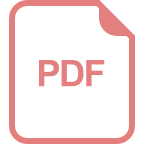





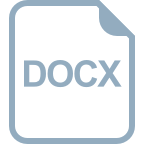
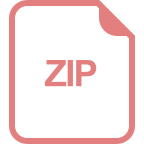
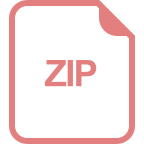
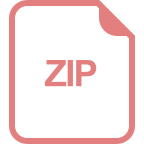
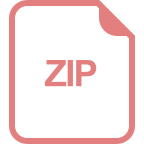
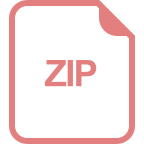