std::string 如何使用
时间: 2024-09-10 12:30:50 浏览: 55
`std::string` 是 C++ 标准库中的一个类,用于表示字符串。它是动态大小的,可以根据需要自动调整内存大小。以下是使用 `std::string` 的基本步骤:
1. **包含头文件**:
首先要在代码中包含 `<string>` 头文件:
```cpp
#include <string>
```
2. **创建字符串对象**:
可以通过构造函数、赋值运算符或直接初始化的方式创建 `std::string` 对象,例如:
- 构造函数:
```cpp
std::string str1 = "Hello, World!";
```
- 赋值运算符:
```cpp
std::string str2;
str2 = "Another string";
```
- 初始化列表:
```cpp
std::string str3{"A third string"};
```
3. **访问和修改字符串内容**:
- 使用 `at(index)` 或 `[index]` 访问单个字符,注意索引从0开始:
```cpp
char firstChar = str1[0];
```
- 使用 `substr(start, length)` 获取子串:
```cpp
std::string substr = str1.substr(7, 5); // "World"
```
- 修改字符:
```cpp
str2[0] = 'H'; // 改变第二个字符
```
4. **字符串操作**:
- `length()`: 返回字符串长度:
```cpp
size_t len = str3.length();
```
- `append(str)`: 追加字符串:
```cpp
str1.append(" is great!");
```
- `find(str, pos=0)`: 查找子串的位置,如果没有找到则返回 `npos`:
```cpp
size_t foundPos = str1.find("World");
```
5. **释放资源**:
`std::string` 自动管理其内存,所以无需显式释放。当对象生命周期结束时,内存会被自动回收。
相关推荐
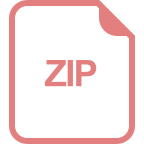
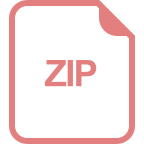
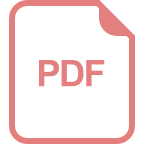















