c# socket服务端
时间: 2024-08-28 16:02:01 浏览: 21
在C#中,Socket服务端是通过System.Net套接字类来创建网络通信的基础。Socket代表了一个网络连接点,它允许程序发送和接收数据。以下是建立一个基本的TCP Socket服务器的基本步骤:
1. **创建Socket实例**:
使用`Socket`类的构造函数创建一个新的TCP套接字,例如`TcpListener serverSocket = new TcpListener(IPAddress.Any, port)`,这里`IPAddress.Any`表示监听所有可用的IP地址,`port`是你想要使用的端口号。
2. **开始监听**:
调用`StartListening()`方法开启监听,服务器会等待客户端的连接请求。
3. **接受连接**:
使用`AcceptSocket()`方法接收一个新的连接请求,这将返回一个新的`Socket`实例,用于与客户端通信。
4. **处理连接**:
循环读取并处理客户端发送的消息,可以使用`Receive`方法接收数据,并根据需要编写相应的响应。
5. **关闭连接**:
当完成通信后,记得关闭连接,即`client.Close()`。
下面是一个简单的示例代码片段:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class Program
{
static void Main()
{
TcpListener listener = new TcpListener(IPAddress.Any, 12345);
listener.Start();
Console.WriteLine("Server is listening on port {0}", listener.LocalEndPoint.Port);
while (true)
{
Socket client = listener.AcceptSocket();
Console.WriteLine("Accepted connection from " + client.RemoteEndPoint.ToString());
// Handle the client connection here...
byte[] buffer = new byte[256];
int bytesRead = client.Receive(buffer);
string message = System.Text.Encoding.ASCII.GetString(buffer, 0, bytesRead);
Console.WriteLine("Received: " + message);
// Send a response back to the client...
byte[] response = Encoding.ASCII.GetBytes("Hello, Client!");
client.Send(response, 0, response.Length);
client.Close(); // Close the connection after handling it
}
}
}
```
相关推荐
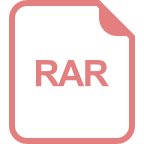
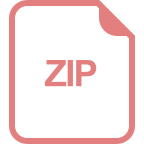














