#include<stdio.h> #include<time.h> #define SIZE 11 typedef struct{ int key; }rectype; int Partition(rectype N[],int m,int n) { int i,j; rectype temp; i=m; j=n; temp=N[i]; do { while((N[j].key>=temp.key)&&(i<j)) j--; if(i<j) N[i++] = N[j]; while((N[i].key<=temp.key) && (i<j)) i++; if(i<j) N[j--] = N[i]; }while(i != j); N[i] = temp; return i; } void QuickSort(rectype N[], int s1, int t1) { int i; if(s1 < t1) { i = Partition(R, s1, t1); QuickSort(R, s1, i-1); QuickSort(R, i+1, t1); } } void main() { rectype R[SIZE]; int i; printf("请输入使用快速排序算法排序的10个数据\n"); for(i=1; i < SIZE; i++) { scanf("%d",&R[i].key); } printf("快速排序之前\n"); for(i = 1; i < SIZE; i++) { printf("%d\t",R[i].key); printf("\n"); } QuickSort(R,1,10); printf("快速排序之后\n"); for(i = 1; i < SIZE; i++) { printf("%d\t",R[i].key); } }请加上必要的注释
时间: 2023-06-23 18:47:41 浏览: 70
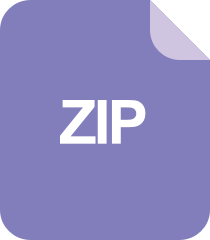
SeqList.h #include <stdio.h> //包含标准输入输出流的头文件 #include <assert.h
注释已添加在代码中,如下所示:
```c
#include<stdio.h>
#include<time.h>
#define SIZE 11
typedef struct{
int key; // 定义结构体,用于存储待排序数据
} rectype;
/* 快速排序算法的划分函数 */
int Partition(rectype N[], int m, int n) {
int i, j;
rectype temp;
i = m;
j = n;
temp = N[i];
do {
/* 右侧指针j向左移动,找到第一个小于temp的元素 */
while ((N[j].key >= temp.key) && (i < j))
j--;
if (i < j)
N[i++] = N[j];
/* 左侧指针i向右移动,找到第一个大于temp的元素 */
while ((N[i].key <= temp.key) && (i < j))
i++;
if (i < j)
N[j--] = N[i];
} while (i != j);
/* 将枢轴元素temp放入最终的位置 */
N[i] = temp;
return i;
}
/* 快速排序算法的递归函数 */
void QuickSort(rectype N[], int s1, int t1) {
int i;
/* 当前子序列的长度小于2时,停止递归 */
if (s1 < t1) {
/* 将当前子序列划分为两个子序列 */
i = Partition(N, s1, t1);
/* 对左侧子序列进行排序 */
QuickSort(N, s1, i-1);
/* 对右侧子序列进行排序 */
QuickSort(N, i+1, t1);
}
}
void main() {
rectype R[SIZE];
int i;
printf("请输入使用快速排序算法排序的10个数据\n");
for (i = 1; i < SIZE; i++) {
scanf("%d", &R[i].key);
}
printf("快速排序之前\n");
for (i = 1; i < SIZE; i++) {
printf("%d\t", R[i].key);
}
printf("\n");
/* 对数据进行快速排序 */
QuickSort(R, 1, 10);
printf("快速排序之后\n");
for (i = 1; i < SIZE; i++) {
printf("%d\t", R[i].key);
}
printf("\n");
}
```
阅读全文
相关推荐
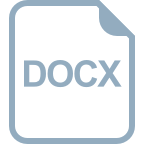
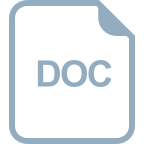
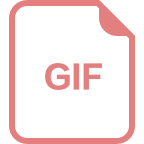
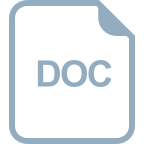
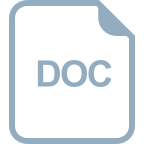
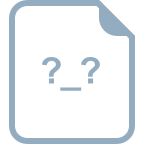


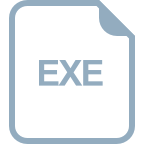
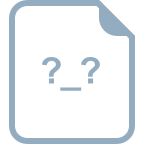
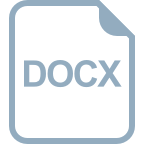
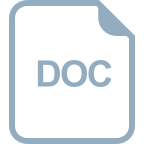
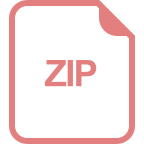