import pandas as pd import numpy as np from matplotlib import pyplot as plt #2.读取数据 from sklearn.metrics import f1_score from sklearn.model_selection import train_test_split from sklearn.tree import DecisionTreeClassifier data=pd.read_excel('train_data.xlsx') #print(data) #print(np.any(data.isnull())) #3.数据预处理 data=data.drop(labels=['机器编号','统一规范代码','具体故障类别'],axis=1)#删除不需要的列 data.replace('L',1.0,inplace=True) data.replace('M',2.0,inplace=True) data.replace('H',3.0,inplace=True) data=data.dropna() #print(data) X = data.iloc[:,0:-1] y = data.iloc[:,-1] #4、分割测试集和训练集 X_train,X_test,y_train,y_test = train_test_split(X,y,test_size=0.33,random_state=40) #5、选择模型 model = DecisionTreeClassifier(max_depth=6) #6、训练模型 model.fit(X_train,y_train) #7、评价模型:要求用F1 #y_predict = model.predict(X_test) #score = f1_score(y_predict,y_test,average="macro") #plt.show() y_predict = model.predict(X_test) score = f1_score(y_predict,y_test,average="macro") print(score) 补全缺失值填充
时间: 2024-03-13 20:47:46 浏览: 202
在数据预处理的步骤中,可以对缺失值进行填充。常见的缺失值填充方法包括均值填充、中位数填充、众数填充、前向填充、后向填充、插值填充等。具体采用哪种方法,需要根据数据的具体情况和分析目的来决定。
在这份代码中,可以使用以下方法对缺失值进行填充:
1. 均值填充
```python
data.fillna(data.mean(), inplace=True)
```
2. 中位数填充
```python
data.fillna(data.median(), inplace=True)
```
3. 众数填充
```python
data.fillna(data.mode().iloc[0], inplace=True)
```
其中,`fillna()` 方法可以用来进行填充,`inplace=True` 表示在原始数据上进行修改。如果需要使用新的数据,可以将其赋值给一个新的变量,例如:
```python
new_data = data.fillna(data.mean())
```
相关问题
import pandas as pd import os from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score # 计算准确性 import numpy as np from sklearn.tree import plot_tree import matplotlib.pyplot as plt # 画图 from sklearn.metrics import confusion_matrix # 绘制混淆矩阵时使用 from sklearn.metrics import roc_curve, auc # 绘制ROC曲线时使用 from sklearn.preprocessing import label_binarize # 使用sklearn中的LabelBinarizer可以将多分类标签转化为二分类标签 from sklearn.preprocessing import LabelEncoder from sklearn.preprocessing import LabelBinarizer
您的代码看起来像是在使用Python中的一些流行的机器学习库,如Pandas、Scikit-learn和Numpy。通过这些库,您可以进行数据处理、训练和评估机器学习模型。
具体来说,您的代码中使用了以下功能:
- 导入了pandas、os、sklearn等库;
- 使用sklearn中的train_test_split函数将数据集分为训练集和测试集;
- 使用RandomForestClassifier(随机森林分类器)进行分类任务;
- 使用accuracy_score函数计算模型的准确度;
- 导入numpy库;
- 使用plot_tree函数绘制决策树;
- 使用confusion_matrix函数绘制混淆矩阵;
- 使用roc_curve和auc函数绘制ROC曲线;
- 使用LabelEncoder和LabelBinarizer函数将标签进行编码。
总的来说,这些功能可以帮助您构建和评估机器学习模型。
优化这段代码# import modules 导入模块 from sklearn.model_selection import train_test_split from sklearn.decomposition import PCA import pandas as pd from sklearn import svm import numpy as np import math import matplotlib.pyplot as plt import matplotlib as mpl from matplotlib import colors from sklearn.model_selection import train_test_split from sklearn import datasets from matplotlib.colors import ListedColormap from sklearn.svm import SVC from sklearn.preprocessing import StandardScaler from sklearn.model_selection import StratifiedShuffleSplit,StratifiedKFold from sklearn.model_selection import GridSearchCV from sklearn.model_selection import GridSearchCV, LeaveOneOut, cross_val_predict from sklearn.model_selection import KFold from sklearn.linear_model import LogisticRegression from sklearn.naive_bayes import GaussianNB from sklearn.neighbors import KNeighborsClassifier from sklearn import svm from sklearn.tree import DecisionTreeClassifier from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import roc_auc_score from sklearn.metrics import roc_auc_score import math import datetime import multiprocessing as mp from sklearn.ensemble import StackingClassifier from sklearn.pipeline import make_pipeline from sklearn.svm import LinearSVC import random
你可以对这段代码进行如下优化:
```python
import pandas as pd
import numpy as np
import math
import matplotlib.pyplot as plt
import matplotlib as mpl
from matplotlib import colors
from matplotlib.colors import ListedColormap
from sklearn.model_selection import train_test_split, StratifiedShuffleSplit, StratifiedKFold, GridSearchCV, LeaveOneOut, cross_val_predict, KFold
from sklearn.decomposition import PCA
from sklearn.svm import SVC, LinearSVC
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn.naive_bayes import GaussianNB
from sklearn.neighbors import KNeighborsClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier, StackingClassifier
from sklearn.metrics import roc_auc_score
# 导入模块
# 设置随机种子
random.seed(42)
np.random.seed(42)
# 其他代码...
```
这样做的优点是将导入的模块进行整理和分类,提高了代码的可读性。同时,设置了随机种子,保证了代码的可复现性。你可以根据需要添加其他的代码或模块。
阅读全文
相关推荐
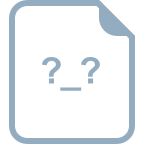
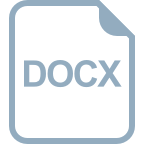
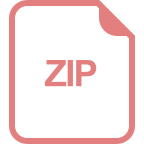














