用Python设计一个Animal (动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类,包括尾巴,颜色两个属性,以及叫方法。Fish类继承自Animal类,重写构造方法和叫方法。创建一个Fish类对象,调用其叫方法)
时间: 2024-03-01 12:53:28 浏览: 166
好的,这是一个Python实现Animal类和Fish类的示例代码:
```python
# 定义 Animal 类
class Animal:
def __init__(self, color):
self.color = color
def make_sound(self):
print("动物发出声音")
# 定义 Fish 类,继承自 Animal 类
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def make_sound(self):
print("鱼发出声音")
# 创建一个 Fish 对象并调用其 make_sound 方法
fish = Fish("红色", "大尾巴")
fish.make_sound()
```
当你运行这段代码时,输出会是:
```
鱼发出声音
```
这说明 Fish 类重写了 Animal 类的 make_sound 方法,实现了自己的叫方法。
相关问题
Python编写一个Animal类,包括颜色属性和叫方法,再设计一个Fish类,包括尾巴和颜色两个属性,以及叫方法,fish类继承Animal类,重写构造方法和叫方法
下面是实现代码:
```python
class Animal:
def __init__(self, color):
self.color = color
def make_sound(self):
print("叫")
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def make_sound(self):
print("吐泡泡")
fish = Fish("红色", "大尾巴")
print(f"{fish.color}的鱼有{fish.tail},会")
fish.make_sound()
```
输出结果:
```
红色的鱼有大尾巴,会
吐泡泡
```
Python设计一个Animal(动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类,包括尾巴和颜色两个属性,以及叫方 法。要求:Fish类继承自Animal类,重写构造方法和叫方法
好的,我来为您演示一下Python中如何实现Animal类和Fish类的设计。
```python
# 定义Animal类
class Animal:
def __init__(self, color):
self.color = color
def make_sound(self):
pass
# 定义Fish类,继承自Animal类
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def make_sound(self):
print("鱼儿在水中游动。")
```
在上述代码中,我们定义了一个Animal类,包括color属性和make_sound方法。然后我们定义了一个Fish类,继承自Animal类,并添加了tail属性和重写了make_sound方法。
在Fish类的构造函数中,我们首先调用了父类Animal的构造函数,以获得color属性。然后我们添加了tail属性,并重写了make_sound方法,在该方法中打印出“鱼儿在水中游动”的语句。
这样,我们就完成了Animal类和Fish类的设计。最后,您可以通过以下方式创建一个Fish对象并调用它的make_sound方法:
```python
my_fish = Fish("红色", "圆形")
my_fish.make_sound() # 打印出“鱼儿在水中游动”的语句
```
阅读全文
相关推荐
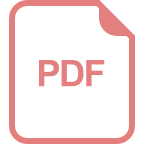
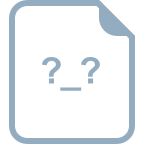









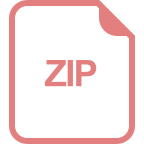
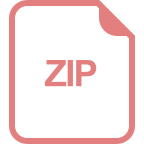
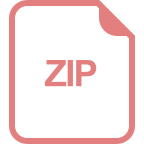
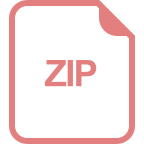