设计一个 Animal(动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类, 包括尾巴和颜色两个属性,以及叫方法。要求:Fish 类继承自 Animal 类,重写构造方法和叫方法
时间: 2024-02-18 19:05:55 浏览: 70
好的,以下是 Animal 和 Fish 类的实现代码:
```python
class Animal:
def __init__(self, color):
self.color = color
def make_sound(self):
pass
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def make_sound(self):
print("Fish doesn't make sound.")
```
在 Animal 类中,我们定义了一个构造方法 `__init__`,它接收一个参数 `color`,并将其赋值给 `self.color` 属性。另外,我们还定义了一个 `make_sound` 方法,它什么也不做,因为不同的动物会有不同的叫声,我们需要在子类中进行具体实现。
在 Fish 类中,我们使用 `super().__init__(color)` 调用父类的构造方法,并且新增一个属性 `tail`。另外,我们重写了父类的 `make_sound` 方法,因为鱼不会发出声音。
相关问题
设计一个Animal(动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类,包括尾巴、颜色两个属性,以及叫方法。Fish类继承自Animal类,重写构造方法和叫方法。创建一个Fish类对象,调用其叫方法
好的,这是你的问题:设计一个Animal(动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类,包括尾巴、颜色两个属性,以及叫方法。Fish类继承自Animal类,重写构造方法和叫方法。创建一个Fish类对象,调用其叫方法。
以下是代码实现:
```python
class Animal:
def __init__(self, color):
self.color = color
def call(self):
print("This animal is making a sound.")
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def call(self):
print("This fish is making a sound by blowing bubbles.")
fish = Fish("blue", "sharp")
fish.call()
```
输出结果为:
```
This fish is making a sound by blowing bubbles.
```
注意,Fish类继承自Animal类,使用`super()`函数调用父类的构造方法,以便在创建Fish对象时初始化color属性。Fish类重写了call()方法,以便在调用时输出鱼的特定叫声。最后,创建了一个Fish对象,调用了它的call()方法,打印出了鱼的叫声。
设计一个Animal(动物)类,包括颜色属性和叫方法。再设计一个Fish(鱼)类,包括尾巴、颜色两个属性,以及叫方法。Fish类继承自Animal类,重写构造方法和叫方法。创建一个Fish类对象,调用其叫方法。
好的,以下是实现代码:
```
class Animal:
def __init__(self, color):
self.color = color
def call(self):
print("动物发出声音")
class Fish(Animal):
def __init__(self, tail, color):
super().__init__(color)
self.tail = tail
def call(self):
print("鱼发出声音")
# 创建Fish类对象
fish_obj = Fish('大尾巴', '红色')
fish_obj.call() # 调用叫方法
```
输出结果为:
```
鱼发出声音
```
阅读全文
相关推荐
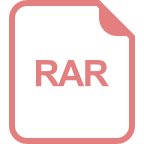
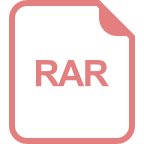







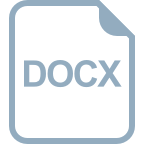
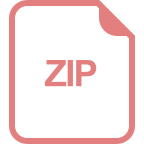