C语言中如何实现健值对
时间: 2024-10-28 17:07:48 浏览: 6
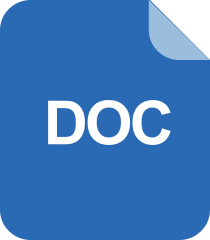
基于单片机实现红外测温仪设计.doc
在C语言中,不像Python那样有内置的数据结构,但可以使用结构体(struct)和关联数组(类似于哈希表)来实现类似键值对的功能。以下是一个基本的实现例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h> // 使用strdup函数复制字符串
// 定义一个结构体用于存储键值对
typedef struct KeyValuePair {
char* key; // 键
int value; // 值
struct KeyValuePair* next; // 链接指向下一个键值对
} KeyValuePair;
// 自定义哈希函数,这里简单地取字符串长度作为哈希值
unsigned int hash(char* key, int table_size) {
return strlen(key);
}
// 哈希表的结点
typedef struct HashTableNode {
KeyValuePair* data;
struct HashTableNode* next;
} HashTableNode;
// 哈希表
typedef struct HashTable {
HashTableNode** table; // 指向键值对的链表
int size;
int numEntries;
} HashTable;
// 创建哈希表
HashTable* createHashTable(int size) {
HashTable* hashTable = (HashTable*) malloc(sizeof(HashTable));
hashTable->table = (HashTableNode**) malloc(size * sizeof(HashTableNode*));
for (int i = 0; i < size; i++) {
hashTable->table[i] = NULL;
}
hashTable->size = size;
hashTable->numEntries = 0;
return hashTable;
}
// 插入键值对
void insertKeyValuePair(HashTable* hashTable, char* key, int value) {
int index = hash(key, hashTable->size);
HashTableNode* newNode = (HashTableNode*) malloc(sizeof(HashTableNode));
newNode->data = (KeyValuePair*) malloc(sizeof(KeyValuePair));
newNode->data->key = strdup(key);
newNode->data->value = value;
newNode->next = hashTable->table[index];
hashTable->table[index] = newNode;
hashTable->numEntries++;
}
// 打印所有键值对
void printHashTable(HashTable* hashTable) {
for (int i = 0; i < hashTable->size; i++) {
HashTableNode* node = hashTable->table[i];
while (node != NULL) {
printf("Key: %s, Value: %d\n", node->data->key, node->data->value);
node = node->next;
}
}
}
int main() {
HashTable* myDict = createHashTable(10);
insertKeyValuePair(myDict, "name", 42);
printHashTable(myDict);
// 清理内存
freeKeyValuePair(myDict, "name");
free(myDict->table);
free(myDict);
return 0;
}
// 辅助函数,用于清理单个键值对
void freeKeyValuePair(HashTable* hashTable, char* key) {
for (int i = 0; i < hashTable->size; i++) {
if (hashTable->table[i]->data && strcmp(hashTable->table[i]->data->key, key) == 0) {
free(hashTable->table[i]->data->key);
free(hashTable->table[i]->data);
hashTable->table[i]->data = NULL;
break;
}
}
}
```
以上代码演示了如何创建和操作一个简单的哈希表实现键值对,注意实际项目中可能需要处理更复杂的情况,如冲突避免和内存管理。
阅读全文
相关推荐
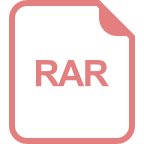
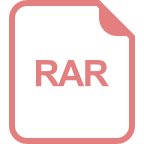
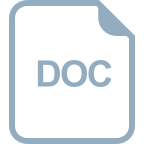
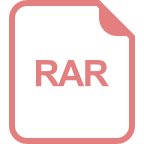
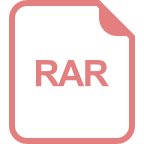
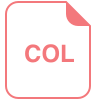
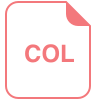
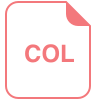
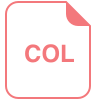
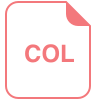
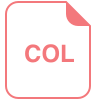
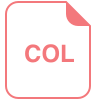
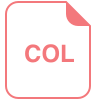
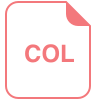
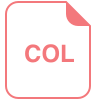
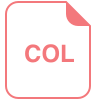
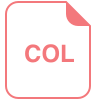