MSP430单片机C语言在物联网应用中的实践:传感器、通信、云平台的完整指南
发布时间: 2024-07-08 20:02:32 阅读量: 37 订阅数: 46 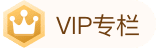
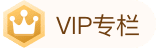
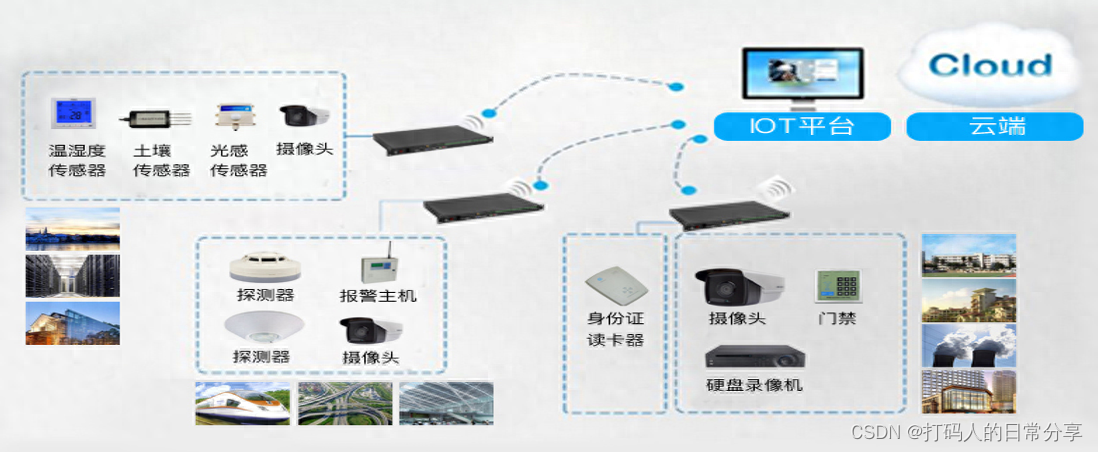
# 1. MSP430单片机C语言简介
MSP430单片机是一种低功耗、高性能的16位混合信号微控制器,广泛应用于嵌入式系统中。其C语言编程具有以下特点:
- **高效性:**C语言代码编译后体积小,运行速度快,适合资源受限的嵌入式系统。
- **可移植性:**C语言代码可以在不同的MSP430型号和编译器之间移植,方便代码复用和维护。
- **丰富的库函数:**MSP430单片机提供丰富的库函数,简化了外设和功能的访问,降低了编程难度。
# 2. MSP430单片机C语言传感器应用
### 2.1 传感器的基本原理和类型
传感器是一种将物理量转换为电信号的装置,广泛应用于工业、农业、医疗、环境监测等领域。MSP430单片机具有低功耗、高性能的特点,非常适合传感器应用。
**2.1.1 温度传感器**
温度传感器是将温度转换为电信号的传感器,常用的类型有热敏电阻、热电偶和半导体温度传感器。
* **热敏电阻:**热敏电阻是一种随着温度变化而改变电阻值的电阻器。其阻值与温度呈负相关关系,温度越高,阻值越小。
* **热电偶:**热电偶是一种由两种不同金属导体连接而成的装置。当两端温度不同时,会在导体之间产生热电势,其大小与温度差成正比。
* **半导体温度传感器:**半导体温度传感器利用半导体的温度特性来测量温度。其内部集成了一个二极管或三极管,其正向导通压降或基极-发射极电压随温度变化而变化。
**2.1.2 湿度传感器**
湿度传感器是将湿度转换为电信号的传感器,常用的类型有电容式湿度传感器、电阻式湿度传感器和光学式湿度传感器。
* **电容式湿度传感器:**电容式湿度传感器利用湿度对电容值的影响来测量湿度。其内部有两个电极,当湿度变化时,电极之间的电容值也会发生变化。
* **电阻式湿度传感器:**电阻式湿度传感器利用湿度对电阻值的影响来测量湿度。其内部有一个吸湿材料,当湿度变化时,吸湿材料的电阻值也会发生变化。
* **光学式湿度传感器:**光学式湿度传感器利用湿度对光吸收率的影响来测量湿度。其内部有一个光源和一个光电探测器,当湿度变化时,光源发出的光被吸湿材料吸收的程度也会发生变化。
**2.1.3 光照传感器**
光照传感器是将光照强度转换为电信号的传感器,常用的类型有光电二极管、光电三极管和光电阻。
* **光电二极管:**光电二极管是一种当受到光照时会产生电流的半导体器件。其电流与光照强度成正比。
* **光电三极管:**光电三极管是一种光电二极管与一个三极管组合而成的器件。其输出电流与光照强度成正比,并且具有放大作用。
* **光电阻:**光电阻是一种随着光照强度变化而改变电阻值的电阻器。其阻值与光照强度成反比,光照强度越大,阻值越小。
### 2.2 MSP430单片机C语言传感器接口编程
MSP430单片机可以通过模拟接口和数字接口与传感器连接。
**2.2.1 模拟传感器接口编程**
模拟传感器接口编程主要涉及模拟数字转换器(ADC)的使用。ADC将模拟信号转换为数字信号,以便单片机可以处理。
```c
#include <msp430.h>
void main() {
// 初始化ADC
ADC10CTL0 = ADC10SHT_2 + ADC10ON;
ADC10CTL1 = ADC10SHP;
ADC10AE0 |= BIT0; // 设置P1.0为ADC输入引脚
while (1) {
// 启动ADC转换
ADC10CTL0 |= ADC10SC;
// 等待转换完成
while (ADC10CTL1 & ADC10BUSY);
// 读取转换结果
uint16_t adcValue = ADC10MEM;
}
}
```
**逻辑分析:**
* 初始化ADC:设置ADC控制寄存器ADC10CTL0和ADC10CTL1,启用ADC并设置采样时间。
* 设置P1.0为ADC输入引脚:将ADC10AE0寄存器中的对应位设置为1。
* 启动ADC转换:将ADC10CTL0寄存器中的ADC10SC位设置为1。
* 等待转换完成:轮询ADC10CTL1寄存器中的ADC10BUSY位,直到其为0。
* 读取转换结果:从ADC10MEM寄存器中读取转换结果。
**2.2.2 数字传感器接口编程**
数字传感器接口编程主要涉及通用输入/输出(GPIO)的使用。GPIO可以配置为输入或输出,以便与传感器进行通信。
```c
#include <msp430.h>
void main() {
// 设置P1.0为输入引脚
P1DIR &= ~BIT0;
P1REN |= BIT0; // 启用上拉电阻
while (1) {
// 读取P1.0的状态
if (P1IN & BIT0) {
// 传感器处于高电平
} else {
// 传感器处于低电平
}
}
}
```
**逻辑分析:**
* 设置P1.0为输入引脚:将P1DIR寄存器中的对应位设置为0,并启用上拉电阻。
* 读取P1.0的状态:轮询P1IN寄存器中的对应位,判断其为高电平还是低电平。
# 3. MSP430单片机C语言通信应用
### 3.1 通信协议和标准
通信协议是通信设备之间交换信息
0
0
相关推荐
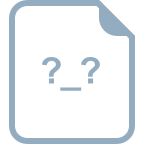
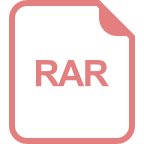
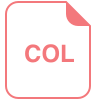
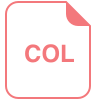
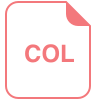



