MSP430单片机C语言数组与指针:数据结构与内存管理实战
发布时间: 2024-07-08 19:06:27 阅读量: 58 订阅数: 30 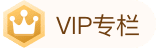
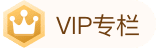
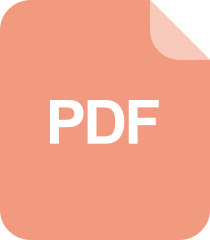
msp430单片机c语言设计
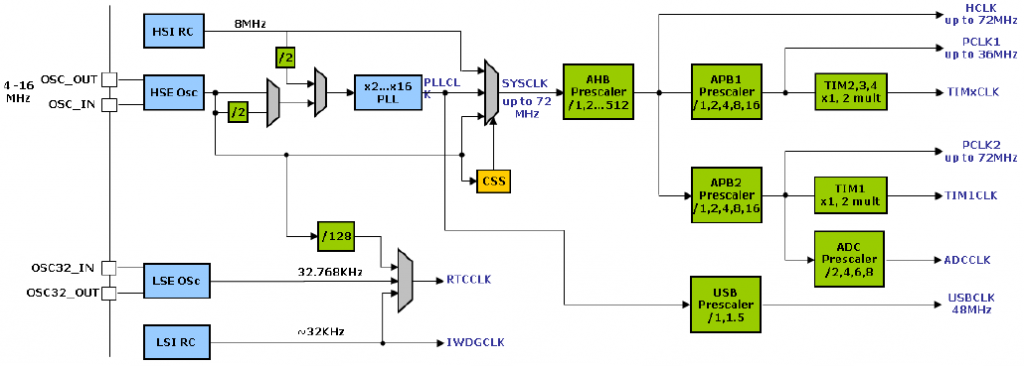
# 1. 数组与指针基础**
数组是一种数据结构,用于存储相同类型的一组元素。指针是一种变量,它存储另一个变量的地址。数组和指针在C语言中广泛用于数据存储和内存管理。
**数组**
数组是一个连续内存区域,用于存储相同类型的数据元素。数组的每个元素都有一个唯一的索引,可以通过索引访问。数组的声明语法如下:
```c
type array_name[size];
```
其中,`type`是数组元素的数据类型,`array_name`是数组的名称,`size`是数组的大小。
**指针**
指针是一个变量,它存储另一个变量的地址。指针的声明语法如下:
```c
type *pointer_name;
```
其中,`type`是指针指向的数据类型,`pointer_name`是指针的名称。
# 2. 数组的应用
数组是一种数据结构,它存储一组具有相同数据类型的元素。数组元素通过索引访问,索引从 0 开始。数组在 C 语言中广泛用于存储和处理数据。
### 2.1 一维数组
#### 2.1.1 数组的定义和初始化
一维数组是一种线性数据结构,它存储一组具有相同数据类型的元素。数组的定义语法如下:
```c
数据类型 数组名[数组大小];
```
例如,定义一个存储 10 个整数的数组:
```c
int numbers[10];
```
数组元素可以使用索引访问,索引从 0 开始。例如,访问数组中的第一个元素:
```c
numbers[0];
```
数组可以使用初始化列表进行初始化。例如,初始化一个存储三个整数的数组:
```c
int numbers[] = {1, 2, 3};
```
#### 2.1.2 数组元素的访问和修改
数组元素可以通过索引访问和修改。例如,访问数组中的第三个元素:
```c
numbers[2];
```
修改数组中的第二个元素:
```c
numbers[1] = 10;
```
### 2.2 多维数组
多维数组是一种存储具有多个维度的元素的数据结构。多维数组的定义语法如下:
```c
数据类型 数组名[维度1][维度2] ... [维度n];
```
例如,定义一个存储 3 行 4 列的二维数组:
```c
int matrix[3][4];
```
多维数组元素可以使用嵌套索引访问。例如,访问二维数组中的 (1, 2) 元素:
```c
matrix[1][2];
```
多维数组也可以使用初始化列表进行初始化。例如,初始化一个存储 2 行 3 列的二维数组:
```c
int matrix[][3] = {
{1, 2, 3},
{4, 5, 6}
};
```
**代码块:**
```c
// 定义一个存储 10 个整数的一维数组
int numbers[10];
// 访问数组中的第一个元素
int first_element = numbers[0];
// 修改数组中的第二个元素
numbers[1] = 10;
// 定义一个存储 3 行 4 列的二维数组
int matrix[3][4];
// 访问二维数组中的 (1, 2) 元素
int matrix_element = matrix[1][2];
```
**逻辑分析:**
* 一维数组 `numbers` 存储 10 个整数,第一个元素的索引为 0。
* 二维数组 `matrix` 存储 3 行 4 列的整数,(1, 2) 元素位于第二行第三列。
**参数说明:**
* `numbers[index]`: 一维数组 `numbers` 中索引为 `index` 的元素。
* `matrix[row][column]`: 二维数组 `matrix` 中位于第 `row` 行第 `column` 列的元素。
# 3.1 指针的基本概念
#### 3.1.1 指针的定义和使用
指针是一种特殊类型的变量,它存储的是另一个变量的地址。通过指针,我们可以间接访问该变量的内容。指针的定义语法如下:
```c
数据类型 *指针名;
```
例如:
```c
int *p;
```
声明了一个指向整数变量的指针 p。
要获取指针指向的变量的值,可以使用解引用运算符 *。例如:
```c
int x = 10;
int *p = &x;
printf("%d\n", *p); // 输出 10
```
#### 3.1.2 指针的运算
指针可以进行以下运算:
- **取地址运算符 &**:获取变量的地址。
- **解引用运算符 ***:获取指针指向的变量的值。
- **加法和减法运算**:指针可以与整数相加或相减,结果是指向新地址的指针。
- **比较运算**:指针可以比较大小或相等性。
例如:
```c
int x = 10;
int *p = &x;
// 取地址
int *q = &p;
// 解引用
printf("%d\n", *p); // 输出 10
// 加法
p++; // 指向下一个整数
// 减法
p--; // 指向前一个整数
// 比较
if (p == q) {
// p 和 q 指向同一个变量
}
```
### 3.2 指针与数组
#### 3.2.1 指针访问数组元素
数组名本身就是一个指向数组首元素的常量指针。因此,我们可以使用指针运算来访问数组元素。
例如:
```c
int arr[] = {1, 2, 3, 4, 5};
// 指向数组首元素
int *p = arr;
// 访问数组元素
printf("%d\n", *p); // 输出 1
printf("%d\n", *(p + 1)); // 输出 2
printf("%d\n", *(p + 2)); // 输出 3
```
#### 3.2.2 数组名作为指针
数组名本身是一个指向数组首元素的常量指针。因此,我们可以将数组名直接赋给指针变量。
例如:
```c
int arr[] = {1, 2, 3, 4, 5};
int *p = arr;
// 访问数组元素
printf("%d\n", *p); // 输出 1
printf("%d\n", *(p + 1)); // 输出 2
printf("%d\n", *(p + 2)); // 输出 3
```
**注意:**数组名作为指针时,指向的是数组的首元素,不能改变。
# 4. 数据结构在MSP430中的应用
### 4.1 链表
**4.1.1 链表的定义和实现**
链表是一种非连续的线性数据结构,其元素通过指针连接起来。每个链表元素通常包含两个部分:数据域和指针域。数据域存储元素的值,而指针域指向下一个元素。
```c
typedef struct node {
int data;
struct node *next;
} node_t;
```
**4.1.2 链表的插入和删除操作**
**插入操作:**
```c
void insert_node(node_t **head, int data) {
node_t *new_node = malloc(sizeof(node_t));
new_node->data = data;
new_node->next = *head;
*head = new_node;
}
```
**删除操作:**
```c
void delete_node(node_t **head, int data) {
node_t *current = *head;
node_t *prev = NULL;
while (current != NULL && current->data != data) {
prev = current;
current = current->next;
}
if (current == NULL) {
return;
}
if (prev == NULL) {
*head = current->next;
} else {
prev->next = current->next;
}
free(current);
}
```
### 4.2 栈
**4.2.1 栈的定义和实现**
栈是一种后进先出(LIFO)的数据结构。元素按顺序添加到栈顶,并从栈顶删除。
```c
typedef struct stack {
int *data;
int top;
int size;
} stack_t;
```
**4.2.2 栈的压入和弹出操作**
**压入操作:**
```c
void push(stack_t *stack, int data) {
if (stack->top == stack->size - 1) {
stack->data = realloc(stack->data, 2 * stack->size * sizeof(int));
stack->size *= 2;
}
stack->data[++stack->top] = data;
}
```
**弹出操作:**
```c
int pop(stack_t *stack) {
if (stack->top == -1) {
return -1; // 栈为空
}
return stack->data[stack->top--];
}
```
### 4.3 队列
**4.3.1 队列的定义和实现**
队列是一种先进先出(FIFO)的数据结构。元素按顺序添加到队列尾部,并从队列头部删除。
```c
typedef struct queue {
int *data;
int front;
int rear;
int size;
} queue_t;
```
**4.3.2 队列的入队和出队操作**
**入队操作:**
```c
void enqueue(queue_t *queue, int data) {
if (queue->rear == queue->size - 1) {
queue->data = realloc(queue->data, 2 * queue->size * sizeof(int));
queue->size *= 2;
}
queue->data[queue->rear++] = data;
}
```
**出队操作:**
```c
int dequeue(queue_t *queue) {
if (queue->front == queue->rear) {
return -1; // 队列为空
}
return queue->data[queue->front++];
}
```
# 5. 内存管理在MSP430中的实战
### 5.1 MSP430的内存结构
MSP430单片机的内存结构主要包括以下几个部分:
- **寄存器组:**寄存器组是CPU内部的存储单元,用于存储程序指令和数据。寄存器组的大小和数量因不同的MSP430型号而异。
- **RAM(随机存取存储器):**RAM是可读写的存储器,用于存储程序代码和数据。RAM的大小也因不同的MSP430型号而异。
- **ROM(只读存储器):**ROM是不可写的存储器,用于存储固件和引导程序。ROM的大小通常是固定的。
### 5.2 内存分配策略
MSP430的内存分配策略主要有两种:
- **静态内存分配:**静态内存分配是在编译时进行的,即编译器会根据程序代码和数据的大小分配固定的内存空间。静态内存分配的优点是执行速度快,但缺点是内存利用率较低。
- **动态内存分配:**动态内存分配是在程序运行时进行的,即程序可以在运行时根据需要分配和释放内存空间。动态内存分配的优点是内存利用率高,但缺点是执行速度比静态内存分配慢。
### 5.3 内存管理函数
MSP430提供了以下几个内存管理函数:
- **malloc()和free()函数:**malloc()函数用于分配动态内存空间,free()函数用于释放动态内存空间。
- **calloc()和realloc()函数:**calloc()函数用于分配并初始化动态内存空间,realloc()函数用于重新分配动态内存空间。
**代码示例:**
```c
#include <stdlib.h>
int main() {
int *p = (int *)malloc(sizeof(int) * 10);
if (p == NULL) {
// 内存分配失败
}
// 使用动态分配的内存空间
free(p);
return 0;
}
```
0
0
相关推荐
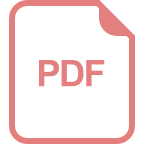
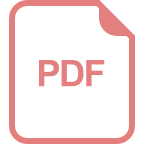
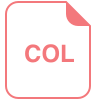
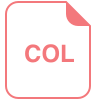
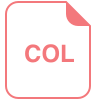
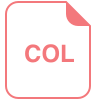
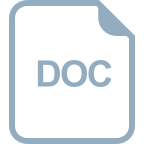
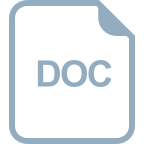