java远程连接linux服务器获取以下信息,1、cpu当前使用率,2、cpu平均使用率,3、内存使用率,4、关键文件系统使用率,5、通过条件判断系统是否正常运行
时间: 2023-07-10 10:06:26 浏览: 253
可以使用Java SSH库(如JSch)来连接Linux服务器,然后执行命令获取所需信息。以下是一个示例代码:
```java
import com.jcraft.jsch.*;
public class SSHClient {
private String host;
private String username;
private String password;
private Session session;
public SSHClient(String host, String username, String password) {
this.host = host;
this.username = username;
this.password = password;
}
public void connect() throws JSchException {
JSch jsch = new JSch();
session = jsch.getSession(username, host, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
}
public void disconnect() {
session.disconnect();
}
public double getCurrentCPUUsage() throws JSchException, InterruptedException {
String command = "top -b -n1 | grep \"Cpu(s)\" | awk '{print $2 + $4}'";
return executeCommandAndGetResult(command);
}
public double getAverageCPUUsage() throws JSchException, InterruptedException {
String command = "top -b -n2 | grep \"Cpu(s)\" | tail -n 1 | awk '{print $2 + $4}'";
return executeCommandAndGetResult(command);
}
public double getMemoryUsage() throws JSchException, InterruptedException {
String command = "free | grep Mem | awk '{print $3/$2 * 100.0}'";
return executeCommandAndGetResult(command);
}
public double getFileSystemUsage(String mountPoint) throws JSchException, InterruptedException {
String command = "df -h " + mountPoint + " | tail -n1 | awk '{print $5}' | sed 's/%//'";
return executeCommandAndGetResult(command);
}
public boolean isSystemRunningNormally() throws JSchException, InterruptedException {
double cpuUsage = getCurrentCPUUsage();
double memoryUsage = getMemoryUsage();
double fileSystemUsage = getFileSystemUsage("/");
return cpuUsage < 80.0 && memoryUsage < 80.0 && fileSystemUsage < 80.0;
}
private double executeCommandAndGetResult(String command) throws JSchException, InterruptedException {
ChannelExec channel = (ChannelExec) session.openChannel("exec");
channel.setCommand(command);
channel.connect();
Thread.sleep(1000);
String result = readInputStream(channel);
channel.disconnect();
return Double.parseDouble(result.trim());
}
private String readInputStream(ChannelExec channel) throws JSchException {
StringBuilder sb = new StringBuilder();
try (java.io.InputStream in = channel.getInputStream()) {
int bufferSize = 1024;
byte[] buffer = new byte[bufferSize];
while (true) {
while (in.available() > 0) {
int bytesRead = in.read(buffer, 0, bufferSize);
sb.append(new String(buffer, 0, bytesRead));
}
if (channel.isClosed()) {
if (in.available() > 0) continue;
break;
}
Thread.sleep(1000);
}
} catch (java.io.IOException e) {
throw new JSchException(e.getMessage(), e);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return sb.toString();
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
SSHClient sshClient = new SSHClient("192.168.1.100", "username", "password");
try {
sshClient.connect();
double currentCPUUsage = sshClient.getCurrentCPUUsage();
double averageCPUUsage = sshClient.getAverageCPUUsage();
double memoryUsage = sshClient.getMemoryUsage();
double fileSystemUsage = sshClient.getFileSystemUsage("/");
boolean isSystemRunningNormally = sshClient.isSystemRunningNormally();
System.out.println("Current CPU usage: " + currentCPUUsage + "%");
System.out.println("Average CPU usage: " + averageCPUUsage + "%");
System.out.println("Memory usage: " + memoryUsage + "%");
System.out.println("File system usage: " + fileSystemUsage + "%");
System.out.println("Is system running normally? " + isSystemRunningNormally);
} catch (JSchException | InterruptedException e) {
e.printStackTrace();
} finally {
sshClient.disconnect();
}
}
}
```
阅读全文
相关推荐
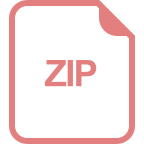
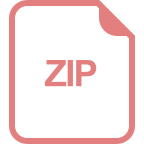
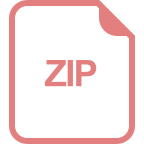
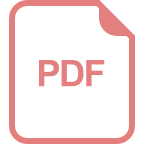
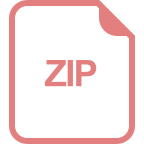
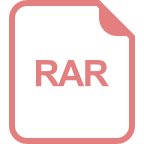
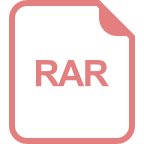
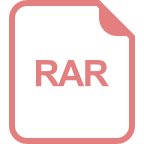
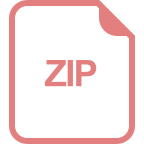
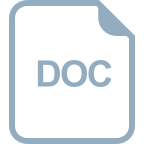
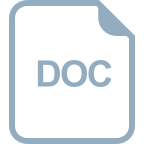
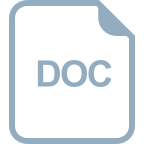
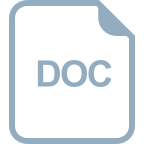
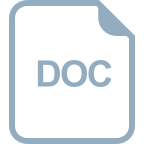
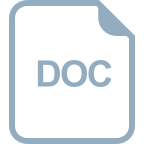