python在列表中间添加元素
时间: 2023-01-11 18:55:36 浏览: 102
在列表中间添加元素可以使用列表的 insert() 方法。例如,如果要在列表的第 2 个位置添加元素 123,可以这样做:
```
lst = [1, 2, 3, 4]
lst.insert(1, 123)
print(lst) # 输出 [1, 123, 2, 3, 4]
```
也可以使用列表切片赋值的方式来插入元素。例如,上面的例子也可以这样写:
```
lst = [1, 2, 3, 4]
lst[1:1] = [123]
print(lst) # 输出 [1, 123, 2, 3, 4]
```
相关问题
python列表中间添加元素
以下是在Python列表中间添加元素的两种方法:
1. 使用insert()方法在指定位置插入元素
```python
list1 = [1, 2, 3, 4]
list1.insert(2, 'a') # 在索引为2的位置插入元素'a'
print(list1) # 输出:[1, 2, 'a', 3, 4]
```
2. 使用切片的方式在指定位置插入元素
```python
list1 = [1, 2, 3, 4]
list1[2:2] = ['a'] # 在索引为2的位置插入元素'a'
print(list1) # 输出:[1, 2, 'a', 3, 4]
```
python 字典中间添加元素
在python中,可以用字典(dict)来存储数据,字典是一种以键值对形式存储数据的数据类型。如果我们需要向字典中添加元素,可以使用以下方法:
1. 直接访问字典中不存在的键,然后将其赋值即可。例如:
```
dict1 = {'Name': 'Alice', 'Age': 20}
dict1['Gender'] = 'Female'
print(dict1)
```
输出结果为:{'Name': 'Alice', 'Age': 20, 'Gender': 'Female'}
2. 使用update()方法,可以将一个字典的键值对添加到另一个字典中。例如:
```
dict1 = {'Name': 'Alice', 'Age': 20}
dict2 = {'Gender': 'Female'}
dict1.update(dict2)
print(dict1)
```
输出结果为:{'Name': 'Alice', 'Age': 20, 'Gender': 'Female'}
3. 使用setdefault()方法,可以向字典中添加键值对,如果该键已存在,则不会修改原有的值。例如:
```
dict1 = {'Name': 'Alice', 'Age': 20}
dict1.setdefault('Gender', 'Female')
dict1.setdefault('Age', 25)
print(dict1)
```
输出结果为:{'Name': 'Alice', 'Age': 20, 'Gender': 'Female'}
综上所述,Python中向字典中添加元素有多种方法,可以根据具体情况选择适合的方法来实现。
阅读全文
相关推荐
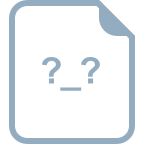



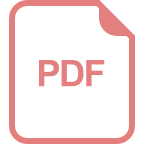
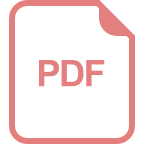
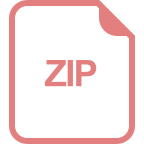
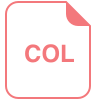
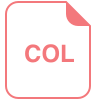
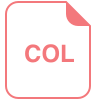




