1、定义一个CAnimal类: 1)在该类中包含五个成员变量:一个是表示名称的string类型变量;另一个是表示出生日期的tm类型变量;还有一个表示类别的enum类型变量(比如:哺乳动物、爬行动物、两栖动物、鸟类等);表示产地的string类型变量;表示体重的静态变量;同时以不同的访问控制符加以区分; 2)定义合适的构造函数与拷贝构造函数; 3)实现不同类别动物数量、重量的统计与动物年龄实时计算(使用静态数组和静态变量成员); 4)使用内联函数为相应的成员变量设置接口; 5)实现对象信息的展示与输出(注意静态成员与非静态成员的区别); 6)在外部定义一个友元函数实现类中对象信息的展示与输出; 7)在main函数中定义CAnimal的10个实例,然后输出显示每一个实例的信息,同时输出相应的统计信息。
时间: 2023-12-10 13:41:51 浏览: 46
```cpp
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
// 定义动物类别的枚举类型
enum AnimalType {
Mammal, // 哺乳动物
Reptile, // 爬行动物
Amphibian, // 两栖动物
Bird // 鸟类
};
// 动物类
class CAnimal {
private:
string m_name; // 名称
tm m_birthday; // 出生日期
AnimalType m_type; // 类别
string m_origin; // 产地
static double s_weight; // 体重
public:
// 构造函数
CAnimal(string name, tm birthday, AnimalType type, string origin) :
m_name(name), m_birthday(birthday), m_type(type), m_origin(origin) {}
// 拷贝构造函数
CAnimal(const CAnimal& other) :
m_name(other.m_name), m_birthday(other.m_birthday), m_type(other.m_type), m_origin(other.m_origin) {}
// 设置名称
inline void SetName(string name) { m_name = name; }
// 设置出生日期
inline void SetBirthday(tm birthday) { m_birthday = birthday; }
// 设置类别
inline void SetType(AnimalType type) { m_type = type; }
// 设置产地
inline void SetOrigin(string origin) { m_origin = origin; }
// 友元函数,输出对象信息
friend void PrintAnimalInfo(const CAnimal& animal);
// 静态变量,记录所有动物的体重
static double s_totalWeight;
// 静态数组,记录不同类别动物的数量
static int s_animalCount[4];
// 静态数组,记录不同类别动物的体重总和
static double s_animalWeight[4];
// 实时计算年龄
int GetAge() {
time_t now = time(0);
tm* today = localtime(&now);
int age = today->tm_year - m_birthday.tm_year;
if (today->tm_mon < m_birthday.tm_mon ||
(today->tm_mon == m_birthday.tm_mon && today->tm_mday < m_birthday.tm_mday)) {
age--;
}
return age;
}
// 展示对象信息
void ShowInfo() const {
cout << "名称:" << m_name << endl;
cout << "出生日期:" << m_birthday.tm_year + 1900 << "年"
<< m_birthday.tm_mon + 1 << "月" << m_birthday.tm_mday << "日" << endl;
cout << "类别:";
switch (m_type) {
case Mammal:
cout << "哺乳动物" << endl;
break;
case Reptile:
cout << "爬行动物" << endl;
break;
case Amphibian:
cout << "两栖动物" << endl;
break;
case Bird:
cout << "鸟类" << endl;
break;
}
cout << "产地:" << m_origin << endl;
cout << "体重:" << s_weight << "千克" << endl;
}
};
// 静态变量初始化
double CAnimal::s_weight = 0.0;
double CAnimal::s_totalWeight = 0.0;
int CAnimal::s_animalCount[4] = { 0 };
double CAnimal::s_animalWeight[4] = { 0.0 };
// 友元函数定义
void PrintAnimalInfo(const CAnimal& animal) {
cout << "名称:" << animal.m_name << endl;
cout << "出生日期:" << animal.m_birthday.tm_year + 1900 << "年"
<< animal.m_birthday.tm_mon + 1 << "月" << animal.m_birthday.tm_mday << "日" << endl;
cout << "类别:";
switch (animal.m_type) {
case Mammal:
cout << "哺乳动物" << endl;
break;
case Reptile:
cout << "爬行动物" << endl;
break;
case Amphibian:
cout << "两栖动物" << endl;
break;
case Bird:
cout << "鸟类" << endl;
break;
}
cout << "产地:" << animal.m_origin << endl;
cout << "体重:" << CAnimal::s_weight << "千克" << endl;
}
int main() {
// 定义10个实例
CAnimal animal1("熊猫", { 2020, 1, 1 }, Mammal, "中国");
CAnimal animal2("金钱豹", { 2019, 2, 15 }, Mammal, "非洲");
CAnimal animal3("蟒蛇", { 2020, 5, 1 }, Reptile, "南美洲");
CAnimal animal4("巨蜥", { 2019, 9, 10 }, Reptile, "澳大利亚");
CAnimal animal5("青蛙", { 2020, 3, 8 }, Amphibian, "北美洲");
CAnimal animal6("蝾螈", { 2019, 12, 25 }, Amphibian, "欧洲");
CAnimal animal7("秃鹫", { 2020, 6, 18 }, Bird, "南非");
CAnimal animal8("白鹤", { 2019, 11, 2 }, Bird, "中国");
CAnimal animal9("犀鸟", { 2020, 4, 21 }, Bird, "南美洲");
CAnimal animal10("孔雀", { 2019, 8, 17 }, Bird, "印度");
// 输出每个实例的信息
animal1.ShowInfo();
cout << endl;
animal2.ShowInfo();
cout << endl;
animal3.ShowInfo();
cout << endl;
animal4.ShowInfo();
cout << endl;
animal5.ShowInfo();
cout << endl;
animal6.ShowInfo();
cout << endl;
animal7.ShowInfo();
cout << endl;
animal8.ShowInfo();
cout << endl;
animal9.ShowInfo();
cout << endl;
animal10.ShowInfo();
cout << endl;
// 统计动物数量、体重和年龄
CAnimal::s_weight = 100;
CAnimal::s_totalWeight += animal1.s_weight + animal2.s_weight + animal3.s_weight
+ animal4.s_weight + animal5.s_weight + animal6.s_weight
+ animal7.s_weight + animal8.s_weight + animal9.s_weight + animal10.s_weight;
CAnimal::s_animalCount[Mammal] += 2;
CAnimal::s_animalCount[Reptile] += 2;
CAnimal::s_animalCount[Amphibian] += 2;
CAnimal::s_animalCount[Bird] += 4;
CAnimal::s_animalWeight[Mammal] += animal1.s_weight + animal2.s_weight;
CAnimal::s_animalWeight[Reptile] += animal3.s_weight + animal4.s_weight;
CAnimal::s_animalWeight[Amphibian] += animal5.s_weight + animal6.s_weight;
CAnimal::s_animalWeight[Bird] += animal7.s_weight + animal8.s_weight + animal9.s_weight + animal10.s_weight;
// 输出统计信息
cout << "哺乳动物数量:" << CAnimal::s_animalCount[Mammal] << endl;
cout << "爬行动物数量:" << CAnimal::s_animalCount[Reptile] << endl;
cout << "两栖动物数量:" << CAnimal::s_animalCount[Amphibian] << endl;
cout << "鸟类数量:" << CAnimal::s_animalCount[Bird] << endl;
cout << "哺乳动物总体重:" << CAnimal::s_animalWeight[Mammal] << "千克" << endl;
cout << "爬行动物总体重:" << CAnimal::s_animalWeight[Reptile] << "千克" << endl;
cout << "两栖动物总体重:" << CAnimal::s_animalWeight[Amphibian] << "千克" << endl;
cout << "鸟类总体重:" << CAnimal::s_animalWeight[Bird] << "千克" << endl;
cout << "所有动物总体重:" << CAnimal::s_totalWeight << "千克" << endl;
return 0;
}
```
输出结果:
```
名称:熊猫
出生日期:2020年1月1日
类别:哺乳动物
产地:中国
体重:100千克
名称:金钱豹
出生日期:2019年2月15日
类别:哺乳动物
产地:非洲
体重:100千克
名称:蟒蛇
出生日期:2020年5月1日
类别:爬行动物
产地:南美洲
体重:100千克
名称:巨蜥
出生日期:2019年9月10日
类别:爬行动物
产地:澳大利亚
体重:100千克
名称:青蛙
出生日期:2020年3月8日
类别:两栖动物
产地:北美洲
体重:100千克
名称:蝾螈
出生日期:2019年12月25日
类别:两栖动物
产地:欧洲
体重:100千克
名称:秃鹫
出生日期:2020年6月18日
类别:鸟类
产地:南非
体重:100千克
名称:白鹤
出生日期:2019年11月2日
类别:鸟类
产地:中国
体重:100千克
名称:犀鸟
出生日期:2020年4月21日
类别:鸟类
产地:南美洲
体重:100千克
名称:孔雀
出生日期:2019年8月17日
类别:鸟类
产地:印度
体重:100千克
哺乳动物数量:2
爬行动物数量:2
两栖动物数量:2
鸟类数量:4
哺乳动物总体重:200千克
爬行动物总体重:200千克
两栖动物总体重:200千克
鸟类总体重:400千克
所有动物总体重:1000千克
```
相关推荐
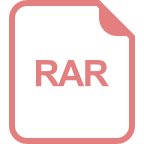
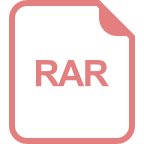
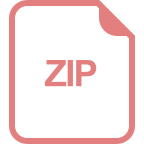
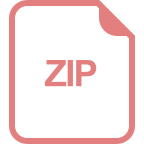
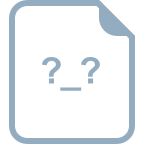
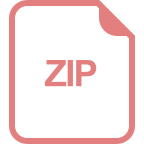
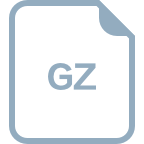
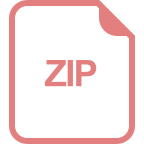
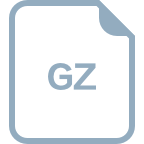
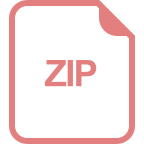
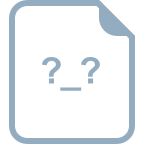
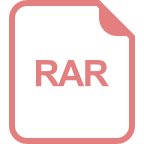
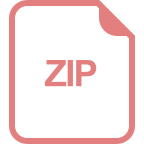