jsch执行exec命令
时间: 2023-10-08 14:14:48 浏览: 83
你可以使用JSch库来连接SSH服务器并执行exec命令。以下是一个简单的示例代码:
```java
import com.jcraft.jsch.*;
public class SSHCommandExecutor {
public static void main(String[] args) {
String host = "your_ssh_host";
String username = "your_ssh_username";
String password = "your_ssh_password";
String command = "your_command_to_execute";
try {
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelExec channelExec = (ChannelExec) session.openChannel("exec");
channelExec.setCommand(command);
channelExec.connect();
InputStream in = channelExec.getInputStream();
byte[] tmp = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(tmp, 0, 1024);
if (i < 0) {
break;
}
System.out.print(new String(tmp, 0, i));
}
if (channelExec.isClosed()) {
if (in.available() > 0) {
continue;
}
System.out.println("exit-status: " + channelExec.getExitStatus());
break;
}
Thread.sleep(1000);
}
channelExec.disconnect();
session.disconnect();
} catch (Exception e) {
System.out.println(e);
}
}
}
```
在这个示例中,我们使用JSch库创建一个连接到SSH服务器的会话,并打开一个exec通道来执行命令。我们通过通道的输入流读取命令的输出,并在控制台上打印出来。最后,我们断开通道和会话连接。
请注意,您需要将“your_ssh_host”、“your_ssh_username”、“your_ssh_password”和“your_command_to_execute”替换为您实际使用的SSH主机、用户名、密码和要执行的命令。
阅读全文
相关推荐
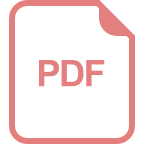










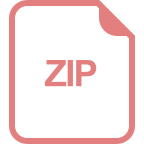
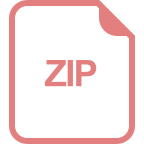
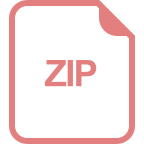

