vue3 ts div中通过flex布局水平放3个子div,其中一个div可以拖拽改变宽度
时间: 2023-08-04 15:20:57 浏览: 63
可以使用 Vue3 + TypeScript + CSS3 实现水平放置3个子 div,其中一个 div 可以拖拽改变宽度的效果。下面是一个简单的实现示例,供你参考:
```html
<template>
<div class="container">
<div class="left" ref="left" :style="{ width: leftWidth + 'px' }">
<!-- 左侧 div,宽度可变 -->
</div>
<div class="middle"></div><!-- 中间 div,固定宽度 -->
<div class="right"></div><!-- 右侧 div,固定宽度 -->
<div class="separator" ref="separator" @mousedown="startDrag"></div><!-- 分隔符,用于拖拽 -->
</div>
</template>
<script lang="ts">
import { ref } from 'vue';
export default {
setup() {
const leftWidth = ref(200); // 左侧 div 的初始宽度
const startDrag = (e: MouseEvent) => {
const separator = e.target as HTMLElement; // 获取分隔符元素
const container = separator.parentElement as HTMLElement; // 获取容器元素
const left = container.querySelector('.left') as HTMLElement; // 获取左侧 div 元素
const startX = e.clientX; // 记录鼠标初始位置
const startWidth = left.offsetWidth; // 记录左侧 div 初始宽度
const onMouseMove = (e: MouseEvent) => {
const diff = e.clientX - startX; // 计算鼠标移动距离
leftWidth.value = startWidth + diff; // 更新左侧 div 宽度
};
const onMouseUp = () => {
document.removeEventListener('mousemove', onMouseMove);
document.removeEventListener('mouseup', onMouseUp);
};
document.addEventListener('mousemove', onMouseMove);
document.addEventListener('mouseup', onMouseUp);
};
return {
leftWidth,
startDrag,
};
},
};
</script>
<style>
.container {
display: flex;
height: 300px;
}
.left {
height: 100%;
background-color: #f0f0f0;
flex-grow: 0;
flex-shrink: 0;
}
.middle {
width: 400px;
height: 100%;
background-color: #e0e0e0;
flex-grow: 0;
flex-shrink: 0;
}
.right {
width: 200px;
height: 100%;
background-color: #d0d0d0;
flex-grow: 0;
flex-shrink: 0;
}
.separator {
width: 10px;
height: 100%;
background-color: #c0c0c0;
flex-grow: 0;
flex-shrink: 0;
cursor: col-resize;
}
</style>
```
在上面的代码中,我们使用了 Vue3 的 `ref` 函数来创建了两个引用类型变量 `leftWidth` 和 `separator`,分别用于记录左侧 div 的宽度和分隔符元素。
在 `startDrag` 函数中,我们首先获取了分隔符、容器和左侧 div 的元素,并记录了鼠标初始位置和左侧 div 初始宽度。然后,我们使用 `addEventListener` 函数添加了 `mousemove` 和 `mouseup` 事件的监听器,分别用于实现拖拽效果。在 `mousemove` 事件中,我们计算了鼠标移动距离,并根据移动距离更新了左侧 div 的宽度。在 `mouseup` 事件中,我们移除了事件监听器,完成了拖拽效果的实现。
最后,我们使用 CSS3 的 flex 布局和一些基本样式,实现了水平放置3个子 div,并在第一个子 div 中添加了拖拽效果。
相关推荐
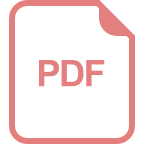
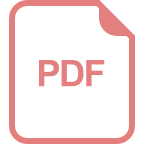














