java+mysql如何显示商品所有信息,显示界面如何写 具体代码
时间: 2024-05-13 17:15:29 浏览: 12
首先,需要连接到MySQL数据库并查询商品数据,可以使用JDBC来实现。以下是一个简单的Java代码示例:
```java
import java.sql.*;
public class DisplayProducts {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/PRODUCTS";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.createStatement();
String sql = "SELECT * FROM products";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
String description = rs.getString("description");
System.out.println("ID: " + id);
System.out.println("Name: " + name);
System.out.println("Price: $" + price);
System.out.println("Description: " + description);
}
rs.close();
stmt.close();
conn.close();
} catch(SQLException se) {
se.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
} finally {
try {
if(stmt!=null) stmt.close();
} catch(SQLException se2) {}
try {
if(conn!=null) conn.close();
} catch(SQLException se) {
se.printStackTrace();
}
}
}
}
```
上述代码通过JDBC连接到名为PRODUCTS的MySQL数据库,查询名为products的表中的所有商品信息,并将其打印到控制台上。
至于显示界面的代码,可以使用Swing或JavaFX等图形用户界面工具包来实现。以下是一个简单的Swing代码示例:
```java
import java.awt.*;
import java.sql.*;
import javax.swing.*;
public class ProductDisplay extends JFrame {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/PRODUCTS";
static final String USER = "username";
static final String PASS = "password";
public ProductDisplay() {
super("Product Display");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(0, 4));
try {
Class.forName(JDBC_DRIVER);
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM products";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
String description = rs.getString("description");
JLabel idLabel = new JLabel("ID: " + id);
JLabel nameLabel = new JLabel("Name: " + name);
JLabel priceLabel = new JLabel("Price: $" + price);
JLabel descriptionLabel = new JLabel("Description: " + description);
add(idLabel);
add(nameLabel);
add(priceLabel);
add(descriptionLabel);
}
rs.close();
stmt.close();
conn.close();
} catch(SQLException se) {
se.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
}
pack();
setVisible(true);
}
public static void main(String[] args) {
new ProductDisplay();
}
}
```
上述代码创建了一个Swing窗口,通过JDBC连接到名为PRODUCTS的MySQL数据库,查询名为products的表中的所有商品信息,并将其显示在窗口中。
相关推荐
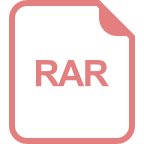
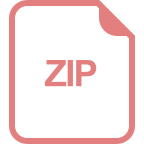
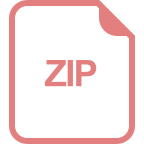














