std::string 转 json 保序
时间: 2024-01-12 11:21:22 浏览: 27
以下是将std::string转换为保序的json的示例代码:
```cpp
#include <iostream>
#include <json/json.h>
std::string JsonAsString(const Json::Value &json) {
std::string result;
Json::StreamWriterBuilder wbuilder;
wbuilder["indentation"] = ""; // 设置缩进为空,保持原始顺序
result = Json::writeString(wbuilder, json);
return result;
}
int main() {
Json::Value rootJsonValue;
rootJsonValue["foo"] = "bar";
std::string jsonString = JsonAsString(rootJsonValue);
std::cout << jsonString << std::endl; // 输出:{"foo":"bar"}
return 0;
}
```
相关问题
帮我写一段将 std::map<std::string,std::string> 转为json 字符串的代码
好的,您可以使用第三方库nlohmann/json来将std::map<std::string,std::string>转为json字符串。具体实现如下:
```c++
#include <iostream>
#include <map>
#include <string>
#include "json.hpp"
using json = nlohmann::json;
int main() {
std::map<std::string, std::string> myMap = {
{"name", "John"},
{"age", "25"},
{"city", "New York"}
};
json j(myMap);
std::string jsonString = j.dump();
std::cout << jsonString << std::endl;
return 0;
}
```
上述代码中,我们首先使用了nlohmann/json库,然后定义了一个std::map<std::string,std::string>类型的变量myMap,并给它赋了一些键值对。接着,我们使用json j(myMap)将myMap转为了json对象j,最后使用j.dump()将json对象转为json字符串。
std::vector 转json
std::vector 转 json 可以使用第三方库,比如JsonCpp。下面是一个示例代码:
```cpp
#include <iostream>
#include <vector>
#include <json/json.h>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
Json::Value jsonValue(Json::arrayValue);
for (const auto& num : numbers) {
jsonValue.append(num);
}
Json::StreamWriterBuilder writer;
std::string jsonString = Json::writeString(writer, jsonValue);
std::cout << jsonString << std::endl;
return 0;
}
```
这段代码将一个 `std::vector<int>` 转换为 JSON 字符串。首先,我们创建一个 `Json::Value` 对象,并将其类型设置为 `arrayValue`,表示它是一个数组。然后,我们遍历 `std::vector` 中的每个元素,将其添加到 `Json::Value` 对象中。最后,我们使用 `Json::StreamWriterBuilder` 和 `Json::writeString` 函数将 `Json::Value` 对象转换为 JSON 字符串。
注意:在使用此示例代码之前,需要先安装 JsonCpp 库,并在编译时链接该库。
相关推荐
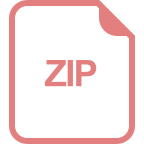













