vue3 中 利用canvas裁剪图片并保存
时间: 2024-02-18 22:12:32 浏览: 234
在 Vue3 中,可以使用以下步骤利用 Canvas 裁剪图片并保存:
1. 在 Vue3 中,可以使用 `ref` 或 `setup()` 中的 `refs` 来获取 `<canvas>` 元素的引用。
```html
<template>
<div>
<canvas ref="canvas"></canvas>
<input type="file" accept="image/*" @change="onFileChange">
<button @click="cropImage">Crop Image</button>
</div>
</template>
```
```javascript
import { ref } from 'vue';
export default {
setup() {
const canvasRef = ref(null);
return {
canvasRef,
};
},
};
```
2. 在 `onFileChange` 方法中获取上传的图片,并将其绘制到 `<canvas>` 中。
```javascript
async function onFileChange(event) {
const file = event.target.files[0];
const image = new Image();
image.onload = () => {
canvasRef.value.width = image.width;
canvasRef.value.height = image.height;
const context = canvasRef.value.getContext('2d');
context.drawImage(image, 0, 0);
};
image.src = URL.createObjectURL(file);
}
```
3. 在 `cropImage` 方法中获取裁剪后的图片,并将其保存。
```javascript
async function cropImage() {
const context = canvasRef.value.getContext('2d');
const imageData = context.getImageData(0, 0, canvasRef.value.width, canvasRef.value.height);
const data = imageData.data;
// 获取裁剪后的图片数据
const croppedImageData = context.getImageData(x, y, width, height);
const croppedData = croppedImageData.data;
// 将裁剪后的图片绘制到新的 Canvas 中
const newCanvas = document.createElement('canvas');
newCanvas.width = width;
newCanvas.height = height;
const newContext = newCanvas.getContext('2d');
newContext.putImageData(croppedImageData, 0, 0);
// 将新的 Canvas 转换为图片,并保存
const newImage = new Image();
newImage.src = newCanvas.toDataURL('image/png');
document.body.appendChild(newImage);
}
```
在以上代码中,`x`、`y`、`width`、`height` 分别表示裁剪区域的左上角坐标和宽高。裁剪后的图片数据可以使用 `getImageData()` 方法获取,然后再将其绘制到新的 Canvas 中,最后将新的 Canvas 转换为图片,并保存。
阅读全文
相关推荐
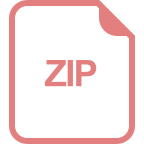
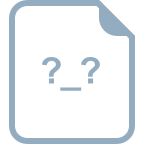
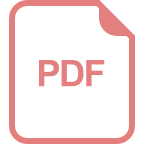
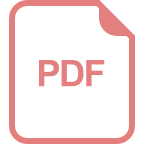
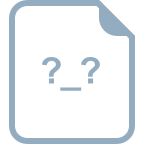
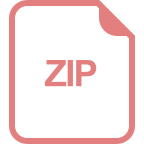
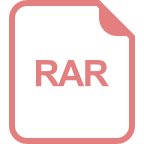
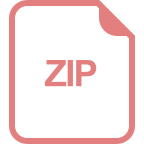
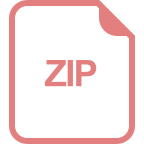
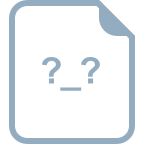
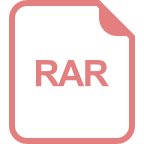
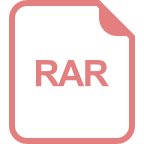
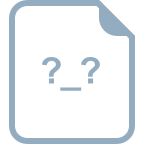
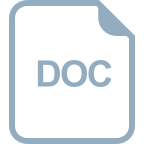
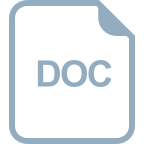
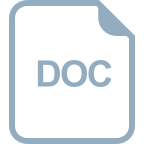
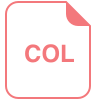