js判断数组是否有重复数据
时间: 2023-08-07 11:03:57 浏览: 44
可以使用Set数据结构来判断数组中是否有重复数据,因为Set只会存储唯一的值。具体做法如下:
```js
function hasDuplicate(arr) {
return new Set(arr).size !== arr.length;
}
// 示例用法
const arr1 = [1, 2, 3, 4];
const arr2 = [1, 2, 3, 1];
console.log(hasDuplicate(arr1)); // false
console.log(hasDuplicate(arr2)); // true
```
另外,也可以使用双重循环来判断数组中是否有重复数据,但是时间复杂度较高,不建议使用:
```js
function hasDuplicate(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j]) {
return true;
}
}
}
return false;
}
```
相关问题
js 判断数组是否有重复的对象元素
好的,您可以使用 Set 数据结构来判断数组是否有重复的对象元素,以下是示例代码:
```javascript
function hasDuplicate(array) {
return (new Set(array)).size !== array.length;
}
// 示例用法
const arr1 = [1, 2, 3, 4, 5]; // 没有重复元素
console.log(hasDuplicate(arr1)); // false
const arr2 = [1, 2, 3, 4, 5, 5]; // 有重复元素 5
console.log(hasDuplicate(arr2)); // true
const arr3 = [{id: 1}, {id: 2}, {id: 3}, {id: 1}]; // 有重复元素 {id: 1}
console.log(hasDuplicate(arr3)); // true
```
希望这个答案能够对您有所帮助,还有什么问题可以继续问我哦。
js 判断数组是否存在重复元素
可以通过以下代码来判断一个数组是否存在重复元素:
```javascript
function hasDuplicates(arr) {
return new Set(arr).size !== arr.length;
}
// 示例:
const arr1 = [1, 2, 3, 4, 5];
console.log(hasDuplicates(arr1)); // false
const arr2 = [1, 1, 2, 3, 4];
console.log(hasDuplicates(arr2)); // true
```
请注意:以上代码是使用 ES6 的 Set 数据结构实现的,因此需要在支持 Set 的环境下运行。如果需要支持不同的环境,可以考虑使用遍历数组的方式判断是否存在重复元素。
相关推荐
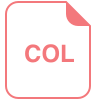
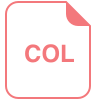
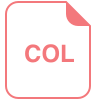
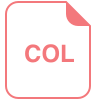
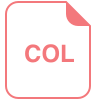







