js byte[]和string转化
时间: 2024-01-05 09:04:17 浏览: 31
在JavaScript中,可以通过以下方式将byte[]转换为string:
1. 使用TextDecoder()构造函数将byte[]转换为string:
```javascript
const decoder = new TextDecoder('utf-8');
const byteArray = new Uint8Array([72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]);
const str = decoder.decode(byteArray);
console.log(str); // Output: Hello World
```
2. 使用String.fromCharCode()方法将byte[]转换为string:
```javascript
const byteArray = new Uint8Array([72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]);
const str = String.fromCharCode.apply(null, byteArray);
console.log(str); // Output: Hello World
```
将string转换为byte[]可以通过以下方式实现:
1. 使用TextEncoder()构造函数将string转换为byte[]:
```javascript
const encoder = new TextEncoder();
const str = 'Hello World';
const byteArray = encoder.encode(str);
console.log(byteArray); // Output: Uint8Array(11) [72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]
```
2. 使用ArrayBuffer()构造函数将string转换为byte[]:
```javascript
const str = 'Hello World';
const buffer = new ArrayBuffer(str.length * 2); // 2 bytes per character
const byteArray = new Uint8Array(buffer);
for (let i = 0; i < str.length; i++) {
byteArray[i] = str.charCodeAt(i);
}
console.log(byteArray); // Output: Uint8Array(22) [72, 0, 101, 0, 108, 0, 108, 0, 111, 0, 32, 0, 87, 0, 111, 0, 114, 0, 108, 0, 100, 0]
```
相关推荐
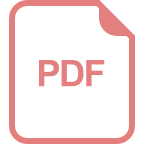
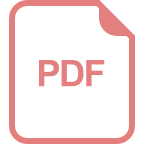
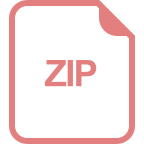















