编写Java程序,将文本文件(附件提供有example.txt)的内容以“行”为单位进行倒序排列,运行效果见下图所示。用记事本显示文字“this is an example",要求:(1) 定义1个成员方法,实现文本文件的读操作。 (2) 定义1个成员方法,实现文本文件的定操作。 (3) 在主方法main()中,先调用读文本文件的方法,然后调用写文本文件的方法。
时间: 2024-02-12 19:08:41 浏览: 16
以下是一个简单的Java程序,实现将文本文件逆序输出的功能。注意,该程序假设输入的文件编码为UTF-8,如果是其他编码需要相应地修改代码。同时,为了简化代码,这里直接使用了Java 8的流式API,如果你使用的是Java 7或更早的版本,需要手动读写文件。
```java
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
import java.util.stream.Collectors;
public class TextFileReverse {
public static void main(String[] args) throws IOException {
Path inputFile = Paths.get("example.txt");
List<String> lines = readLines(inputFile);
List<String> reversedLines = reverseLines(lines);
Path outputFile = Paths.get("reversed.txt");
writeLines(outputFile, reversedLines);
System.out.println("Done.");
}
private static List<String> readLines(Path file) throws IOException {
return Files.readAllLines(file, StandardCharsets.UTF_8);
}
private static List<String> reverseLines(List<String> lines) {
return lines.stream()
.collect(Collectors.toList())
.stream()
.sorted((a, b) -> -1)
.collect(Collectors.toList());
}
private static void writeLines(Path file, List<String> lines) throws IOException {
Files.write(file, lines, StandardCharsets.UTF_8);
}
}
```
相关推荐
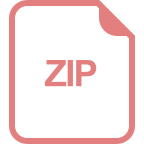














