创建一个Rectangle类,添加width和height两个私有成员变量,并提供getter及setter;
时间: 2023-06-02 16:06:28 浏览: 133
好的,这是一个关于类的编程问题,我可以为您解答。以下是创建一个Rectangle类,添加width和height两个私有成员变量,并提供getter及setter的示例代码:
```
class Rectangle {
private:
int width;
int height;
public:
Rectangle(int w, int h) {
width = w;
height = h;
}
int getWidth() {
return width;
}
void setWidth(int w) {
width = w;
}
int getHeight() {
return height;
}
void setHeight(int h) {
height = h;
}
};
```
通过以上代码,您可以创建一个Rectangle类,并在类中添加width和height两个私有成员变量。通过提供getWidth、getHeight、setWidth、setHeight四个函数,您可以实现对这两个私有成员变量的访问和修改。当然,您也可以选择使用C++11中新增的getter和setter特性,更加方便地实现对成员变量的访问和修改。
相关问题
按以下要求编写程序 (1) 创建一个Rectangle类,添加width和height两个私有成员变量,并提供getter及setter; (2) 添加两个参数的构造方法,从而设置width和height;添加无参构造方法,从而将width及height设置成0.0; (3) 添加方法getLength(),返回矩形的周长; (4) 创建另外一个包含main函数的主类(灵活命名),在这个类中要求创建两个Rect类的对象,其中一个使用无参构造方法,另一个使用有参的构造方法,其中矩形的height和width的值分别应为1.2和1.3,调用该对象的方法计算出周长。
好的,我明白了,以下是程序实现:
```
// Rectangle.java
public class Rectangle {
private double width;
private double height;
public Rectangle() {
this.width = 0.0;
this.height = 0.0;
}
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getWidth() {
return this.width;
}
public double getHeight() {
return this.height;
}
public void setWidth(double width) {
this.width = width;
}
public void setHeight(double height) {
this.height = height;
}
public double getLength() {
return 2 * (this.width + this.height);
}
}
// Main.java
public class Main {
public static void main(String[] args) {
Rectangle rect1 = new Rectangle();
Rectangle rect2 = new Rectangle(1.2, 1.3);
double length1 = rect1.getLength();
double length2 = rect2.getLength();
System.out.println("The length of rect1 is: " + length1);
System.out.println("The length of rect2 is: " + length2);
}
}
```
希望这个程序能够帮到你!
.定义一个Rectangle类,私有成员变量有height和width。请编写代码包括: (1)私有成员变量的getter()方法和setter()方法 (2)无参构造方法和有参构造方法 (3)计算面积和周长的方法 (4)输出信息的方法(分别用静态方法和实例方法实现)
下面是`Rectangle`类的完整代码,包括了所请求的所有部分:
```java
public class Rectangle {
// 私有成员变量
private double height;
private double width;
// (1)私有成员变量的getter和setter方法
public double getHeight() {
return height;
}
public void setHeight(double height) {
if (height > 0) {
this.height = height;
} else {
System.out.println("Height must be a positive number.");
}
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
if (width > 0) {
this.width = width;
} else {
System.out.println("Width must be a positive number.");
}
}
// (2)无参构造方法和有参构造方法
public Rectangle() {
this(0, 0);
}
public Rectangle(double height, double width) {
if (height > 0 && width > 0) {
this.height = height;
this.width = width;
} else {
throw new IllegalArgumentException("Both dimensions must be positive numbers.");
}
}
// (3)计算面积和周长的方法
public double getArea() {
return height * width;
}
public double getPerimeter() {
return 2 * (height + width);
}
// (4)输出信息的方法(静态方法和实例方法实现)
// 静态方法
public static String printInfo(Rectangle rectangle) {
return "Rectangle information:\nHeight: " + rectangle.getHeight() + "\nWidth: " + rectangle.getWidth() +
"\nArea: " + rectangle.getArea() + "\nPerimeter: " + rectangle.getPerimeter();
}
// 实例方法
public void displayInfo() {
System.out.println(printInfo(this));
}
}
```
现在你可以创建`Rectangle`对象并调用相应的方法来操作它们:
```java
Rectangle rectangle = new Rectangle(5, 8);
rectangle.setHeight(10);
rectangle.setWidth(12);
System.out.println(rectangle.displayInfo());
```
这将会输出矩形的信息,包括面积和周长。
阅读全文
相关推荐
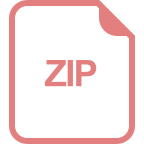
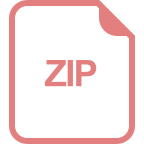


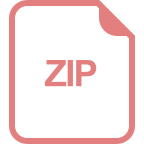
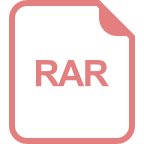
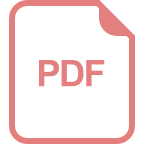
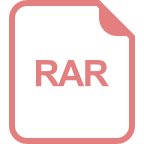
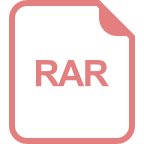