如何用python抓取网页上导出为excel的数据
时间: 2023-05-12 14:05:38 浏览: 480
可以使用 Python 的 requests 库和 pandas 库来抓取网页上导出为 Excel 的数据。具体步骤如下:
1. 使用 requests 库发送 GET 请求获取网页内容。
2. 使用 pandas 库的 read_html 函数将网页内容解析为 DataFrame。
3. 将 DataFrame 导出为 Excel 文件。
下面是示例代码:
```python
import requests
import pandas as pd
url = 'https://example.com/export_excel'
response = requests.get(url)
df_list = pd.read_html(response.text)
df = df_list[0] # 假设第一个表格是需要导出的数据
df.to_excel('data.xlsx', index=False)
```
其中,url 是需要抓取的网页地址,response.text 是网页内容的文本形式,df_list 是解析出的 DataFrame 列表,df 是需要导出的 DataFrame,'data.xlsx' 是导出的 Excel 文件名。
相关问题
帮我写一段可以抓取网页数据并且导出到excel中的代码
当然可以,以下是一个Python的示例代码:
```python
import requests
import pandas as pd
url = 'https://www.example.com' # 替换为你要抓取的网页地址
response = requests.get(url)
data = response.text
# 使用pandas将数据导出到excel中
df = pd.DataFrame({'data': [data]})
df.to_excel('data.xlsx', index=False)
```
这段代码可以抓取指定网页的数据,并将数据导出到名为"data.xlsx"的Excel文件中。你可以根据自己的需求修改代码。
用Python爬虫之抓取 Boss直聘 数据到 Excel 中
在Python中,我们可以使用一些库如BeautifulSoup、requests和pandas来抓取网页数据并将其保存到Excel文件中。以下是抓取Boss直聘这类网站的数据的一个基本步骤:
1. 安装必要的库:
- `pip install beautifulsoup4`:用于解析HTML文档
- `pip install requests`:发送HTTP请求获取网页内容
- `pip install pandas`:处理表格数据并导出到Excel
2. 导入所需的模块:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
```
3. 发送GET请求获取网页源码:
```python
url = 'https://www.zhipin.com/' # 替换为你需要抓取的页面URL
response = requests.get(url)
html_content = response.text
```
4. 使用BeautifulSoup解析HTML:
```python
soup = BeautifulSoup(html_content, 'lxml')
data_rows = soup.find_all('div', class_='job-item') # 根据实际的HTML结构选择合适的元素
```
5. 提取所需数据:
通常,你需要找到每个职位信息所在的特定标签,例如标题、描述、薪资等。这可能涉及到嵌套的选择器,例如:
```python
job_list = []
for row in data_rows:
title = row.find('h3').text.strip() # 提取职位标题
description = row.find('.job-detail').text.strip() # 提取职位描述
# ...继续提取其他字段
job_list.append([title, description, ...]) # 将数据放入列表
```
6. 将数据转化为DataFrame并保存到Excel:
```python
df = pd.DataFrame(job_list, columns=['职位名称', '职位描述', '...']) # 假设列名已知
output_excel = 'boss_zhipin_data.xlsx'
df.to_excel(output_excel, index=False)
```
完成上述步骤后,你应该就能成功地从Boss直聘上抓取数据并保存到Excel文件了。
阅读全文
相关推荐
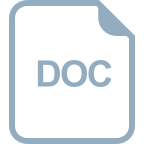
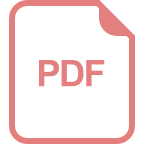
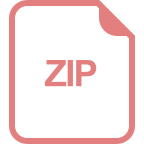
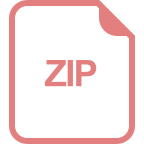
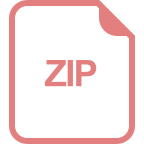
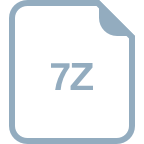
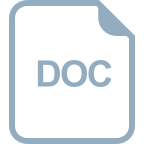
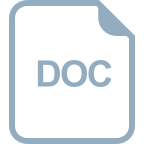
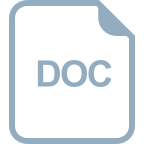
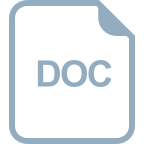





