python 人脸识别 打卡
时间: 2023-11-05 19:05:37 浏览: 108
可以使用Python中的OpenCV库进行人脸识别,具体步骤如下:
1. 安装OpenCV库:可以使用pip install opencv-python命令进行安装。
2. 导入cv2模块:在Python代码中导入cv2模块,该模块包含了OpenCV库的所有函数和类。
3. 加载人脸识别模型:使用cv2.CascadeClassifier()函数加载人脸识别模型,该函数需要传入一个xml文件作为参数,该文件包含了训练好的人脸识别模型。
4. 读取图片并进行灰度化处理:使用cv2.imread()函数读取图片,并使用cv2.cvtColor()函数将图片转换为灰度图像。
5. 进行人脸识别:使用cv2.CascadeClassifier.detectMultiScale()函数进行人脸识别,该函数会返回一个矩形框,表示检测到的人脸位置。
6. 在图片上绘制矩形框:使用cv2.rectangle()函数在图片上绘制矩形框,将检测到的人脸位置标注出来。
7. 显示图片:使用cv2.imshow()函数显示处理后的图片。
相关问题
使用Python编写人脸识别打卡系统
人脸识别打卡系统是在人脸识别系统的基础上,增加了打卡记录、考勤统计等功能。以下是一个简单的人脸识别打卡系统的实现步骤:
1. 收集员工人脸图像数据,并建立人脸识别模型。
2. 在打卡地点安装摄像头,在摄像头拍摄到员工人脸图像时,进行人脸识别,并记录打卡时间和员工信息。
3. 将打卡记录存储到数据库中,并进行考勤统计和分析。
具体实现方案如下:
1. 建立人脸识别模型,可以使用OpenCV等图像处理库和机器学习库,参照上一题的实现步骤。
2. 使用Python的摄像头库,如OpenCV的VideoCapture库,获取摄像头拍摄到的图像,并进行预处理、人脸检测和识别。
3. 将识别结果与员工信息进行匹配,记录打卡时间和员工信息,并将记录存储到数据库中。
4. 在数据库中查询员工的打卡记录,并进行考勤统计和分析,如迟到、早退、加班等情况。
需要注意的是,人脸识别打卡系统需要保证数据安全和隐私保护,遵守相关法规和规定。同时,为了保证识别准确率和效率,可以优化算法和硬件环境,如使用GPU加速、优化人脸检测算法等。
python人脸识别记录员工的打卡信息。
要实现这个功能,你可以使用 Python 中的 OpenCV 库进行人脸识别,然后再将记录员工的打卡信息存储到数据库中。
以下是一个简单的实现步骤:
1. 使用 OpenCV 检测摄像头中的人脸,可以使用 Haar 级联分类器或者深度学习模型进行人脸检测。
2. 对检测到的人脸进行特征提取,可以使用深度学习模型进行特征提取。
3. 将提取到的特征与已有员工信息的特征进行比对,如果匹配成功,则记录员工的打卡信息并存储到数据库中。
4. 在每次打卡时,可以通过摄像头检测到员工的面部特征,然后进行比对,如果匹配成功则记录打卡信息。
需要注意的是,为了保证准确性,需要收集足够的员工特征,并且定期更新员工特征以适应员工面部特征的变化。另外,为了保护员工的隐私,应该严格控制特征的访问权限,并采取必要的数据保护措施。
阅读全文
相关推荐
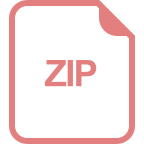
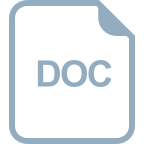
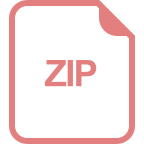
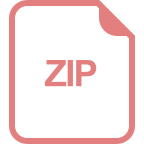
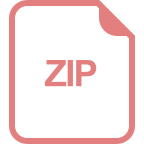
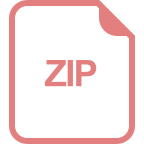
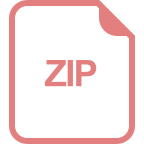
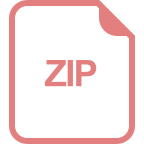
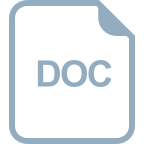
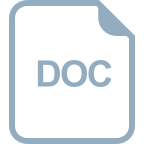

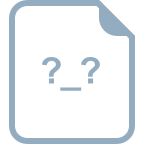
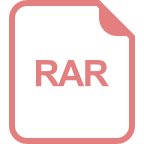
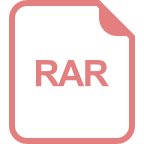
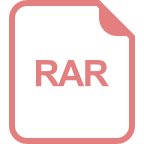