python人脸识别记录员工的打卡信息,代码
时间: 2023-11-22 21:55:11 浏览: 35
这里给你提供一个基于OpenCV和face_recognition库的Python人脸识别记录员工打卡信息的代码:
```python
import cv2
import face_recognition
import numpy as np
import datetime
# 加载已知员工的图片和姓名
known_face_encodings = []
known_face_names = []
image_paths = ['employee1.jpg', 'employee2.jpg', 'employee3.jpg']
for path in image_paths:
image = face_recognition.load_image_file(path)
face_encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(face_encoding)
known_face_names.append(path.split(".")[0])
# 打开摄像头
video_capture = cv2.VideoCapture(0)
while True:
# 捕获一帧图像
ret, frame = video_capture.read()
# 将RGB图像转换为BGR图像以适应OpenCV
rgb_frame = frame[:, :, ::-1]
# 查找当前帧中所有面部和面部编码
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
# 在此帧中循环遍历每个人脸
for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings):
# 看看面部是否与已知的面部相匹配
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
# 如果找到了匹配项,则使用第一个匹配项的姓名作为名称
# 如果找不到匹配项,则使用“未知”作为名称
name = "Unknown"
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
# 在面部周围绘制一个方框
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
# 在面部下方绘制名称
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 1.0, (255, 255, 255), 1)
# 记录员工的打卡信息
if name != "Unknown":
with open("attendance.txt", "a") as f:
f.write(f"{name} {datetime.datetime.now()}\n")
# 显示结果图像
cv2.imshow('Video', frame)
# 按“Q”键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 清理
video_capture.release()
cv2.destroyAllWindows()
```
上述代码首先加载已知员工的图片和姓名,然后打开摄像头进行实时人脸识别。如果找到的人脸与已知的人脸之一匹配,则在图像中标记出人脸并记录员工的打卡信息。最后,代码会在窗口中显示实时识别结果,按下“Q”键退出程序。
相关推荐
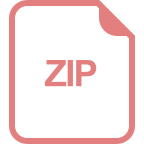
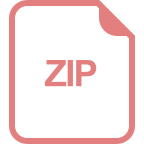














