解决过拟合和欠拟合的代码
时间: 2023-09-23 16:03:30 浏览: 89
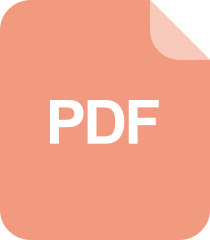
过拟合欠拟合及其解决方案

解决过拟合和欠拟合的方法有很多,下面是一些基本的解决方法和相应的代码实现:
解决过拟合的方法:
1. 数据增强:通过对训练数据进行一些随机变换,增加数据量,使模型不容易记住训练集的特征,从而减少过拟合。
```python
from tensorflow.keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(
rotation_range=20, # 随机旋转角度
width_shift_range=0.2, # 水平方向平移
height_shift_range=0.2, # 垂直方向平移
shear_range=0.2, # 剪切变换
zoom_range=0.2, # 随机缩放
horizontal_flip=True, # 水平翻转
fill_mode='nearest' # 填充方式
)
```
2. 正则化:通过在损失函数中增加正则项,限制模型的复杂度,避免过拟合。
```python
from tensorflow.keras import regularizers
model = Sequential([
Dense(64, activation='relu', kernel_regularizer=regularizers.l2(0.001)),
Dropout(0.5),
Dense(10, activation='softmax')
])
```
3. 提前停止:在训练过程中,当验证集的准确率不再提高时,提前结束训练,避免过拟合。
```python
from tensorflow.keras.callbacks import EarlyStopping
early_stopping = EarlyStopping(monitor='val_acc', patience=5)
model.fit(x_train, y_train, validation_data=(x_val, y_val), epochs=100, callbacks=[early_stopping])
```
解决欠拟合的方法:
1. 增加模型复杂度:增加模型的层数、神经元数量等,提高模型的表达能力,避免欠拟合。
```python
model = Sequential([
Dense(128, activation='relu'),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
```
2. 增加训练次数:增加训练次数,让模型更充分地学习数据特征,避免欠拟合。
```python
model.fit(x_train, y_train, validation_data=(x_val, y_val), epochs=100)
```
3. 特征工程:通过对原始数据进行一些特征变换,提高数据的表达能力,避免欠拟合。
```python
from sklearn.preprocessing import PolynomialFeatures
poly = PolynomialFeatures(degree=2)
x_train_poly = poly.fit_transform(x_train)
x_val_poly = poly.transform(x_val)
model = Sequential([
Dense(64, activation='relu', input_shape=(x_train_poly.shape[1],)),
Dense(10, activation='softmax')
])
model.fit(x_train_poly, y_train, validation_data=(x_val_poly, y_val), epochs=100)
```
阅读全文
相关推荐
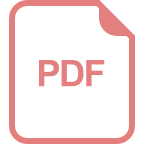
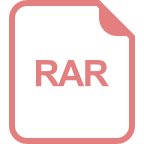

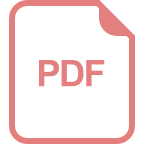
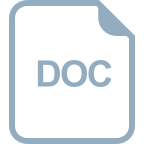
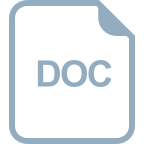
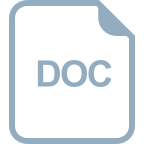
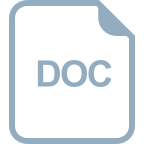
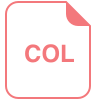
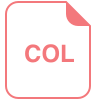
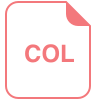
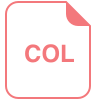
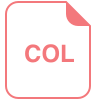
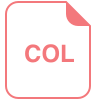
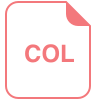
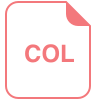
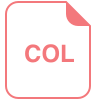
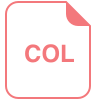