jsp中application和session有什么区别
时间: 2024-01-09 16:04:28 浏览: 253
JSP中的application和session都是用来在web应用程序中保持数据或状态的对象,但它们之间有一些区别。
application对象是全局的,它代表整个web应用程序,在所有JSP页面中都是可见的。它可以用来存储在整个应用程序中共享的数据,比如全局变量。
session对象则是与用户相关联的,它代表了用户的一个会话,可以在用户不同的请求之间保存用户的状态信息。通常,session对象可以用来存储关于用户的信息,比如用户的登录状态、购物车内容等。
总结一下,application对象用于全局共享数据,而session对象用于针对单个用户的状态管理。
相关问题
jsp中session和application的区别
jsp中的session和application都是用来在不同页面之间共享数据的对象,但它们的作用范围有所不同。
session是会话级别的对象,表示在同一个浏览器会话中不同的请求之间共享数据。比如说,在用户登录后,可以将用户的一些信息存放在session对象中,方便在用户浏览网站的不同页面时,能够快速地获取到这些信息。
application是应用级别的对象,表示在整个web应用程序中共享数据。它的生命周期与web应用程序相同,当web应用程序启动时创建,当web应用程序关闭时销毁。比如说,在网站中使用的一些共享数据,如全局配置参数、数据库连接池等,就可以存放在application对象中。
因此,session和application的区别在于它们的作用范围不同,一个是会话级别的对象,一个是应用级别的对象。
用 jsp中的application和session做个用户注册
以下是一个基本的用户注册示例,使用JSP中的application和session:
register.jsp:
```
<html>
<head>
<title>User Registration</title>
</head>
<body>
<h1>User Registration</h1>
<%
String errorMessage = (String) request.getAttribute("errorMessage");
if (errorMessage != null) {
out.println("<p style=\"color:red\">" + errorMessage + "</p>");
}
%>
<form method="post" action="register.do">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="Register">
</form>
</body>
</html>
```
register.do:
```
<%@ page import="java.util.*" %>
<%@ page import="javax.servlet.*" %>
<%@ page import="javax.servlet.http.*" %>
<%
String username = request.getParameter("username");
String password = request.getParameter("password");
// Validate input
if (username == null || username.trim().length() == 0 ||
password == null || password.trim().length() == 0) {
request.setAttribute("errorMessage", "Please enter a username and password");
request.getRequestDispatcher("register.jsp").forward(request, response);
return;
}
// Check if username already exists
ServletContext application = getServletContext();
Map<String, String> users = (Map<String, String>) application.getAttribute("users");
if (users == null) {
users = new HashMap<String, String>();
application.setAttribute("users", users);
}
if (users.containsKey(username)) {
request.setAttribute("errorMessage", "Username already exists");
request.getRequestDispatcher("register.jsp").forward(request, response);
return;
}
// Add user to application scope
users.put(username, password);
// Add user to session scope
HttpSession session = request.getSession();
session.setAttribute("username", username);
response.sendRedirect("welcome.jsp");
%>
```
welcome.jsp:
```
<html>
<head>
<title>Welcome</title>
</head>
<body>
<h1>Welcome <%= session.getAttribute("username") %></h1>
<p>You have successfully registered.</p>
<p><a href="logout.do">Logout</a></p>
</body>
</html>
```
logout.do:
```
<%@ page import="javax.servlet.*" %>
<%@ page import="javax.servlet.http.*" %>
<%
// Invalidate session
HttpSession session = request.getSession(false);
if (session != null) {
session.invalidate();
}
// Redirect to login page
response.sendRedirect("login.jsp");
%>
```
阅读全文
相关推荐
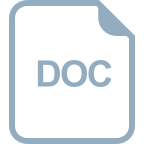
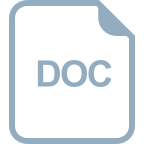
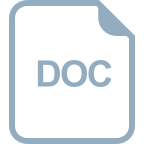
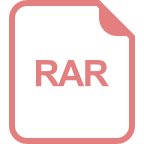
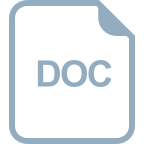
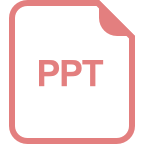
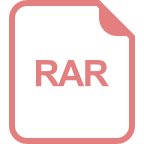
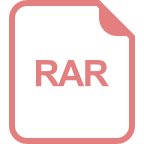
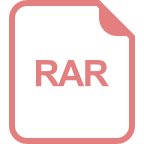
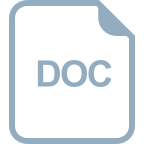
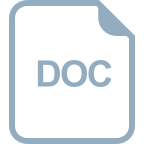
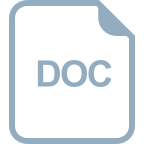




