Service依赖注入举例说明
时间: 2023-07-08 19:54:02 浏览: 47
假设我们正在开发一个电商网站,其中有一个购物车服务,需要依赖一个库存服务来检查商品是否有足够的库存。这时候,我们可以使用依赖注入来解决这个问题。
首先,我们需要定义一个购物车服务的接口,比如:
```
public interface ShoppingCartService {
void addProduct(String productId, int quantity);
boolean checkout();
}
```
然后,我们需要定义一个库存服务的接口,比如:
```
public interface InventoryService {
int getStock(String productId);
}
```
接下来,在购物车服务中,我们可以通过构造函数或者 setter 方法来注入库存服务的实例,比如:
```
public class ShoppingCartServiceImpl implements ShoppingCartService {
private InventoryService inventoryService;
public ShoppingCartServiceImpl(InventoryService inventoryService) {
this.inventoryService = inventoryService;
}
public void addProduct(String productId, int quantity) {
int stock = inventoryService.getStock(productId);
if (quantity <= stock) {
// 添加商品到购物车
} else {
throw new RuntimeException("商品库存不足");
}
}
public boolean checkout() {
// 结算购物车
return true;
}
public void setInventoryService(InventoryService inventoryService) {
this.inventoryService = inventoryService;
}
}
```
这样,在使用购物车服务的时候,我们可以将一个库存服务的实例注入到购物车服务中,比如:
```
InventoryService inventoryService = new InventoryServiceImpl();
ShoppingCartService shoppingCartService = new ShoppingCartServiceImpl(inventoryService);
```
这样,购物车服务就可以使用库存服务来检查商品是否有足够的库存了。这就是一个依赖注入的例子。
相关推荐
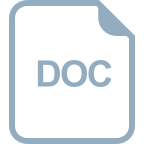
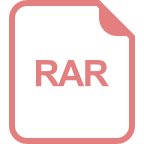
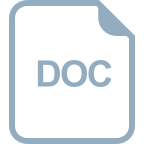














